已知在坐标系中有一个长方形物体,ABCD分别为4个角,已知ABCD4个点的坐标,现在要在另一坐标系中展示该物体,以A为原点,AB为Y轴,AD为X轴,求原坐标到新坐标的转换公式,用java代码实现
时间: 2023-09-03 10:16:39 浏览: 191
假设原坐标系中A(x1, y1),B(x2, y2),C(x3, y3),D(x4, y4)四个点的坐标已知,新坐标系中A(0, 0),B(0, h),D(w, 0)三个点的坐标已知。
首先,我们可以求出AB和AD两个向量:
AB = (0, h) - (0, 0) = (0, h)
AD = (w, 0) - (0, 0) = (w, 0)
然后,我们可以求出四个点相对于A点的坐标:
B' = (x2 - x1, y2 - y1)
C' = (x3 - x1, y3 - y1)
D' = (x4 - x1, y4 - y1)
接着,我们可以将B'和D'分别投影到AB和AD向量上,得到它们在新坐标系中的坐标:
B'' = ((B' · AB) / |AB|^2) * AB = (h * (y2 - y1) / |AB|, h * (y2 - y1) / |AB|)
D'' = ((D' · AD) / |AD|^2) * AD = (w * (x4 - x1) / |AD|, w * (x4 - x1) / |AD|)
最后,我们可以得到C''的坐标,因为C'' = B'' + D'' - A:
C'' = B'' + D'' = (h * (y2 - y1) / |AB| + w * (x4 - x1) / |AD|, h * (y2 - y1) / |AB| + w * (x4 - x1) / |AD|)
Java代码实现如下:
```java
public static double[][] convertCoordinates(double[][] originalCoords, double w, double h) {
double x1 = originalCoords[0][0];
double y1 = originalCoords[0][1];
double x2 = originalCoords[1][0];
double y2 = originalCoords[1][1];
double x3 = originalCoords[2][0];
double y3 = originalCoords[2][1];
double x4 = originalCoords[3][0];
double y4 = originalCoords[3][1];
double[][] newCoords = new double[4][2];
// Calculate AB and AD vectors
double abX = 0;
double abY = h;
double adX = w;
double adY = 0;
// Calculate B', C', and D' coordinates
double bx = x2 - x1;
double by = y2 - y1;
double cx = x3 - x1;
double cy = y3 - y1;
double dx = x4 - x1;
double dy = y4 - y1;
// Calculate projections of B' and D' onto AB and AD vectors
double bProj = (bx * abX + by * abY) / (abX * abX + abY * abY);
double dProj = (dx * adX + dy * adY) / (adX * adX + adY * adY);
// Calculate B'' and D'' coordinates
double bx2 = bProj * abX;
double by2 = bProj * abY;
double dx2 = dProj * adX;
double dy2 = dProj * adY;
// Calculate C'' coordinates
double cx2 = bx2 + dx2;
double cy2 = by2 + dy2;
// Store new coordinates in array
newCoords[0][0] = 0;
newCoords[0][1] = 0;
newCoords[1][0] = bx2;
newCoords[1][1] = by2;
newCoords[2][0] = cx2;
newCoords[2][1] = cy2;
newCoords[3][0] = dx2;
newCoords[3][1] = dy2;
return newCoords;
}
```
阅读全文
相关推荐
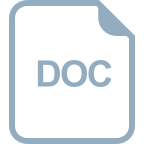
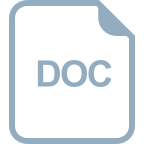
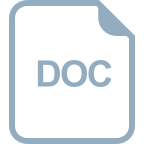















