三个数组分别与另外三个数组对比,有则删除再添加到对应的数组中,无则直接添加到相对于的数组中 js例子
时间: 2024-02-27 13:53:58 浏览: 47
好的,以下是一个简单的JavaScript示例,演示如何将三个数组进行比较,并根据对比结果将元素添加到对应的数组中:
```javascript
// 假设有三个数组a1、a2、a3和对应的数组b1、b2、b3
let a1 = [1, 2, 3];
let a2 = [2, 3, 4];
let a3 = [3, 4, 5];
let b1 = [];
let b2 = [];
let b3 = [];
// 遍历数组a1,将元素添加到对应的数组中
for (let i = 0; i < a1.length; i++) {
// 如果元素在a2和a3中存在,则删除a1中的元素,并添加到b1中
if (a2.includes(a1[i]) && a3.includes(a1[i])) {
let index = a1.indexOf(a1[i]);
a1.splice(index, 1);
b1.push(a1[i]);
}
// 如果元素只在a2中存在,则添加到b2中
else if (a2.includes(a1[i])) {
b2.push(a1[i]);
}
// 如果元素只在a3中存在,则添加到b3中
else if (a3.includes(a1[i])) {
b3.push(a1[i]);
}
}
// 遍历数组a2,将元素添加到对应的数组中
for (let i = 0; i < a2.length; i++) {
// 如果元素在a1和a3中存在,则删除a2中的元素,并添加到b1中
if (a1.includes(a2[i]) && a3.includes(a2[i])) {
let index = a2.indexOf(a2[i]);
a2.splice(index, 1);
b1.push(a2[i]);
}
// 如果元素只在a1中存在,则添加到b2中
else if (a1.includes(a2[i])) {
b2.push(a2[i]);
}
// 如果元素只在a3中存在,则添加到b3中
else if (a3.includes(a2[i])) {
b3.push(a2[i]);
}
}
// 遍历数组a3,将元素添加到对应的数组中
for (let i = 0; i < a3.length; i++) {
// 如果元素在a1和a2中存在,则删除a3中的元素,并添加到b1中
if (a1.includes(a3[i]) && a2.includes(a3[i])) {
let index = a3.indexOf(a3[i]);
a3.splice(index, 1);
b1.push(a3[i]);
}
// 如果元素只在a1中存在,则添加到b2中
else if (a1.includes(a3[i])) {
b2.push(a3[i]);
}
// 如果元素只在a2中存在,则添加到b3中
else if (a2.includes(a3[i])) {
b3.push(a3[i]);
}
}
// 输出结果
console.log("a1:", a1);
console.log("a2:", a2);
console.log("a3:", a3);
console.log("b1:", b1);
console.log("b2:", b2);
console.log("b3:", b3);
```
在上面的示例中,我们先定义了三个数组a1、a2、a3和对应的数组b1、b2、b3。然后,我们使用三个for循环,遍历数组a1、a2、a3中的元素。对于每个元素,我们判断它是否在其他两个数组中存在,如果存在,则从原数组中删除该元素,并将其添加到对应的数组中。如果不存在,则直接将其添加到对应的数组中。最后,我们输出结果,以验证代码是否正确执行。
阅读全文
相关推荐
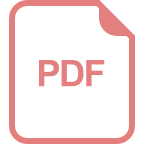
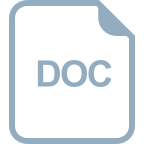
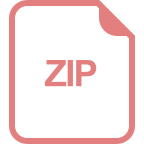
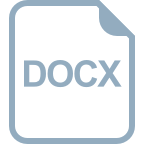
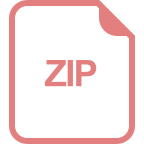
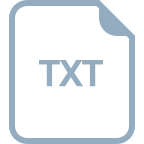
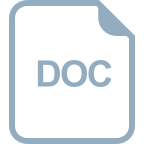
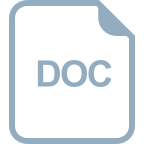
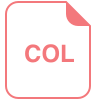
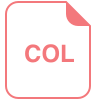
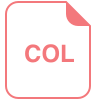
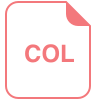
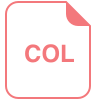
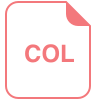
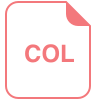
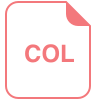