golang发送http请求
时间: 2023-11-25 20:51:45 浏览: 207
以下是两种golang发送http请求的例子:
1.使用http.NewRequest发送请求
```go
import (
"fmt"
"net/http"
"net/url"
)
func main() {
apiUrl := "https://api.example.com/v1/users"
data := url.Values{}
data.Set("name", "John Doe")
data.Set("email", "johndoe@example.com")
req, err := http.NewRequest("POST", apiUrl, strings.NewReader(data.Encode()))
if err != nil {
panic(err)
}
req.Header.Set("Content-Type", "application/x-www-form-urlencoded")
req.Header.Set("Authorization", "Bearer YOUR_ACCESS_TOKEN")
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
fmt.Println(string(body))
}
```
2.使用http.Post发送请求
```go
import (
"fmt"
"net/http"
"strings"
)
func main() {
apiUrl := "https://api.example.com/v1/users"
data := url.Values{}
data.Set("name", "John Doe")
data.Set("email", "johndoe@example.com")
resp, err := http.Post(apiUrl, "application/x-www-form-urlencoded", strings.NewReader(data.Encode()))
if err != nil {
panic(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
fmt.Println(string(body))
}
```
阅读全文
相关推荐
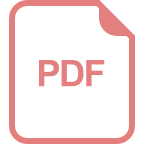
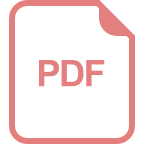
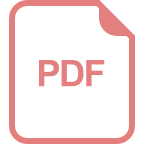


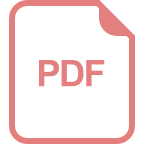


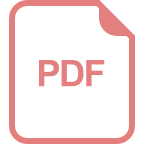
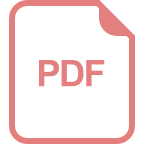
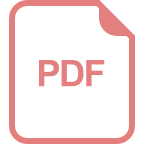
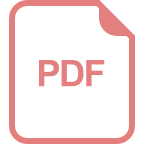
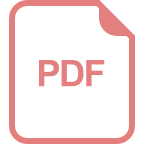



