以英文为例,用计算机穷举的方法破译如下的加法密码密文,CSYEVIXIVQMREXIH用c语言编写一个程序
时间: 2024-09-14 08:08:39 浏览: 70
Sure, I can help you understand how to approach this problem in C language for a simple Caesar Cipher (a type of substitution cipher) decryption using a brute-force method, where we check every possible shift (usually limited to the length of the alphabet) to find the correct key.
First, let's clarify that the given text "CSYEVIXIVQMREXIH" appears to be an English phrase with spaces and capital letters, but for the sake of a basic program, let's assume it's a single string without spaces or case sensitivity, as Caesar Cipher typically deals with consecutive characters shifted by the same amount.
Here's a high-level explanation:
1. Create a loop that iterates through possible shifts from 0 to (the length of your assumed alphabet - 1). In English, the alphabet has 26 letters.
2. For each shift, apply it to each character in the encrypted string. You'll need to convert the characters to their ASCII values and perform the addition modulo 26 (since after 'Z', it wraps back to 'A').
3. Compare the decrypted string with the original text. If they match, you've found the correct key.
4. Since this is a brute force approach, it will take quite some time for larger texts and larger alphabet sizes, so it's not efficient for practical use.
Here's a simple C code snippet to get you started, assuming only lowercase letters and no spaces:
```c
#include <stdio.h>
#include <string.h>
char decryptCaesar(char encrypted[], int shift) {
if ('a' <= encrypted && encrypted <= 'z') {
return ((encrypted - 'a' + shift) % 26) + 'a';
}
// handle other non-letter characters (e.g., punctuation, spaces)
return encrypted;
}
int main() {
char encrypted[] = "csyevixivqmrexih";
const int max_shift = 25; // Assuming English alphabet
for (int i = 0; i <= max_shift; ++i) {
char decrypted[strlen(encrypted)];
for (int j = 0; j < strlen(encrypted); ++j) {
decrypted[j] = decryptCaesar(encrypted[j], i);
}
if (strcmp(decrypted, "your_original_text") == 0) { // Replace with the actual unencrypted text
printf("Decryption key found: %d\n", i);
break; // Stop searching once you find the correct key
}
}
return 0;
}
```
Remember to replace `"your_original_text"` with the plaintext you're trying to recover.
阅读全文
相关推荐
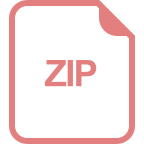
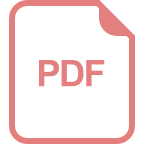



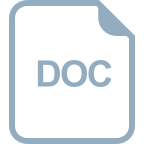
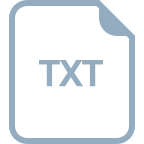
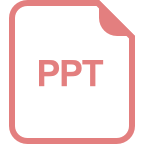
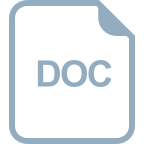
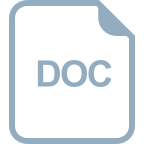
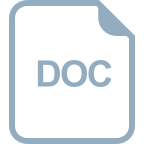






