Vue3代码实现日历组件
时间: 2023-09-06 18:08:16 浏览: 152
以下是一个简单的 Vue3 日历组件的实现示例:
```html
<template>
<div>
<div class="calendar-header">
<button @click="prevMonth">上个月</button>
<h3>{{ monthStr }}</h3>
<button @click="nextMonth">下个月</button>
</div>
<div class="calendar-grid">
<div v-for="(day, index) in days" :key="index" :class="{ 'calendar-cell': true, 'calendar-cell-disabled': !day }">
{{ day }}
</div>
</div>
</div>
</template>
<script>
import { computed, reactive } from 'vue'
export default {
setup() {
const state = reactive({
currentMonth: new Date(),
days: []
})
const prevMonth = () => {
state.currentMonth.setMonth(state.currentMonth.getMonth() - 1)
}
const nextMonth = () => {
state.currentMonth.setMonth(state.currentMonth.getMonth() + 1)
}
const monthStr = computed(() => {
return state.currentMonth.toLocaleString('default', { month: 'long', year: 'numeric' })
})
const generateCalendar = () => {
const firstDay = new Date(state.currentMonth.getFullYear(), state.currentMonth.getMonth(), 1).getDay()
const lastDay = new Date(state.currentMonth.getFullYear(), state.currentMonth.getMonth() + 1, 0).getDate()
const days = new Array(35).fill(null)
for (let i = 1; i <= lastDay; i++) {
days[firstDay + i - 1] = i
}
state.days = days
}
generateCalendar()
return {
state,
prevMonth,
nextMonth,
monthStr
}
}
}
</script>
<style>
.calendar-grid {
display: grid;
grid-template-columns: repeat(7, 1fr);
}
.calendar-cell {
border: 1px solid #ccc;
padding: 10px;
text-align: center;
}
.calendar-cell-disabled {
opacity: 0.3;
}
</style>
```
这个组件使用了 Vue3 的 `reactive` 和 `computed` 函数来声明响应式状态和计算属性。它还使用了 JavaScript 的 `Date` 对象来计算日历表格中的日期。在 `setup` 函数中,我们定义了一些帮助函数来生成日历,并将它们绑定到组件的方法中。最后,我们通过使用 CSS Grid 布局来组织日历表格的每个单元格。
阅读全文
相关推荐
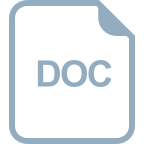
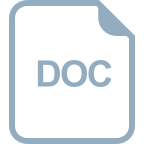
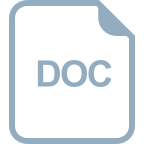
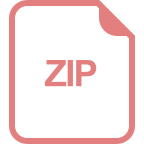
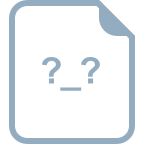
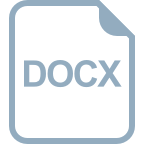
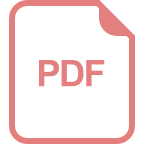
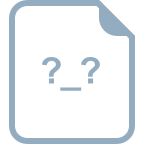
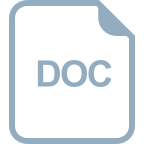
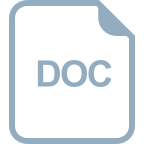
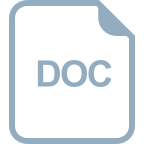






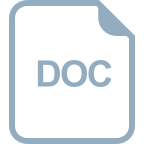