python astra.data3d.get
时间: 2024-05-08 19:04:00 浏览: 24
As an AI language model, I don't have access to the specific library or module you are referring to. However, based on the name of the function, it could potentially be a method within the Astra library for retrieving 3D data. The parameters and return values of the function would depend on the specific implementation within the library.
相关问题
python astra 中fdk算法例子
以下是一个简单的Python Astra FDK算法的例子:
```python
import astra
import numpy as np
import matplotlib.pyplot as plt
# 定义扫描几何体
vol_geom = astra.create_vol_geom(256, 256)
# 定义探测器几何体
det_width = 256
det_size = 256
det_spacing = 1.0
det_angles = np.linspace(0, np.pi, 180, endpoint=False)
det_geom = astra.create_detector_geometry_2d(det_width, det_width, det_size, det_size, det_angles, det_spacing)
# 生成测试数据
data = np.zeros((len(det_angles), det_size))
for i in range(len(det_angles)):
data[i] = np.sin(np.linspace(det_angles[i] - np.pi/2, det_angles[i] + np.pi/2, det_size))
# 创建投影数据
proj_id = astra.create_projector('cuda', det_geom, vol_geom)
sinogram_id, sinogram = astra.create_sino(data, proj_id)
# 创建重建算法
alg_cfg = astra.astra_dict('FDK_CUDA')
alg_cfg['ProjectionDataId'] = sinogram_id
alg_cfg['ReconstructionDataId'] = vol_id
alg_cfg['option'] = {'ShortScan':True}
algorithm_id = astra.algorithm.create(alg_cfg)
# 运行重建算法并获取结果
astra.algorithm.run(algorithm_id)
reconstruction = astra.data2d.get(vol_id)
# 显示重建结果
plt.imshow(reconstruction, cmap='gray')
plt.show()
# 清理内存
astra.algorithm.delete(algorithm_id)
astra.data2d.delete(vol_id)
astra.data2d.delete(sinogram_id)
astra.projector.delete(proj_id)
```
这个例子生成一个$256\times256$的二维正弦图像,并使用Astra库中的FDK算法进行重建。最终结果使用matplotlib库中的imshow函数展示出来。
python astra中FDK
Astra是一个用于CT重建的开源软件库,它支持多种重建算法,包括基于过滤的重建算法(如标准滤波器和扇形滤波器)和迭代重建算法(如SART和MLEM)。其中,FDK(Feldkamp-Davis-Kress)是一种常用的基于过滤的重建算法。
使用Astra进行FDK重建的步骤如下:
1. 导入Astra库
```
import astra
```
2. 定义重建参数
```
# 重建图像的大小
vol_geom = astra.creators.create_vol_geom(nx, ny, nz)
# 探测器的角度范围
angles = np.linspace(0, np.pi, num_angles, False)
# 探测器的数量和每个探测器的像素数
det_width = pixel_size * num_pixels
det_geom = astra.creators.create_detector_geom(detector_size, detector_size, det_width)
# 重建算法的参数
proj_geom = astra.create_proj_geom('cone', pixel_size, pixel_size, num_pixels, num_pixels, angles, source_origin, detector_origin)
proj_id = astra.create_projector('cuda', proj_geom, vol_geom)
recon_id = astra.data2d.create('-vol', vol_geom)
# 过滤器
fpb = 1.0
filter_type = 'ram-lak'
filter_id = astra.create_filter(filter_type, fpb)
# 重建迭代的次数
num_iterations = 10
```
3. 加载投影数据
```
# 投影数据的大小为 num_angles x num_pixels x num_slices
sinogram_id = astra.data2d.create('-sino', proj_geom, data)
```
4. 进行重建
```
# 进行重建
astra.algorithm.run(astra.astra_dict('FDK_CUDA'), sinogram_id, recon_id, filter_id, num_iterations)
# 获取重建图像
recon = astra.data2d.get(recon_id)
```
其中,FDK_CUDA是Astra中用于FDK重建的算法名称,可以根据需要选择其他算法。另外,投影数据需要按照Astra的要求进行格式化,具体可以参考Astra的文档。
需要注意的是,上述代码中使用了CUDA加速,因此需要安装相应的CUDA库并配置好环境。如果没有CUDA环境,可以使用CPU版本的Astra库进行重建。
相关推荐
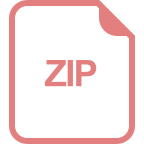












