用以下函数再写一次void InorderTraversal( BinTree BT ); void PreorderTraversal( BinTree BT ); void PostorderTraversal( BinTree BT ); void LevelorderTraversal( BinTree BT );
时间: 2024-03-25 16:38:21 浏览: 89
以下是使用函数实现二叉树的四种遍历方式:
```c++
typedef struct TreeNode *BinTree;
struct TreeNode {
int val;
BinTree left;
BinTree right;
};
// 先序遍历
void PreorderTraversal(BinTree BT) {
if (BT) {
printf("%d ", BT->val);
PreorderTraversal(BT->left);
PreorderTraversal(BT->right);
}
}
// 中序遍历
void InorderTraversal(BinTree BT) {
if (BT) {
InorderTraversal(BT->left);
printf("%d ", BT->val);
InorderTraversal(BT->right);
}
}
// 后序遍历
void PostorderTraversal(BinTree BT) {
if (BT) {
PostorderTraversal(BT->left);
PostorderTraversal(BT->right);
printf("%d ", BT->val);
}
}
// 层序遍历
void LevelorderTraversal(BinTree BT) {
if (BT == NULL) return;
queue<BinTree> q;
q.push(BT);
while (!q.empty()) {
BinTree node = q.front();
q.pop();
printf("%d ", node->val);
if (node->left) q.push(node->left);
if (node->right) q.push(node->right);
}
}
```
其中,先序遍历、中序遍历和后序遍历使用了递归的方式实现,层序遍历使用了队列的数据结构实现。
阅读全文
相关推荐
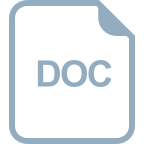
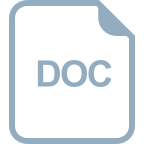
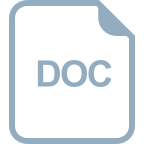
















