结构体类型定义如下: struct stu{ char name[20]; float price; struct stu *next; }; 编写自定义函数创建链表,输入书名和价格,输入Y继续创建,输入其他字符结束创建,编写自定义函数输出最贵的书籍信息,编写自定义函数释放链表内存 输入格式为:请输入书名 价格: \n(循环) 输入格式:"%s %f" 是否继续创建的提示:“是否继续输入,按Y继续输入,其他键结束。\n” 输出提示:“result:\n” 输出格式:“%s %.2f\n”
时间: 2023-11-27 18:49:08 浏览: 73
下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu {
char name[20];
float price;
struct stu *next;
};
// 创建链表
struct stu *createList() {
struct stu *head = NULL;
struct stu *tail = NULL;
char input[10];
do {
struct stu *node = (struct stu*)malloc(sizeof(struct stu));
printf("请输入书名和价格:\n");
scanf("%s %f", node->name, &(node->price));
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
printf("是否继续输入,按Y继续输入,其他键结束。\n");
scanf("%s", input);
} while (strcmp(input, "Y") == 0 || strcmp(input, "y") == 0);
return head;
}
// 输出最贵的书籍信息
void printMax(struct stu *head) {
struct stu *p = head;
struct stu *maxNode = p;
while (p != NULL) {
if (p->price > maxNode->price) {
maxNode = p;
}
p = p->next;
}
printf("result:\n");
printf("%s %.2f\n", maxNode->name, maxNode->price);
}
// 释放链表内存
void freeList(struct stu *head) {
struct stu *p = head;
while (p != NULL) {
struct stu *temp = p;
p = p->next;
free(temp);
}
}
int main() {
struct stu *head = createList();
printMax(head);
freeList(head);
return 0;
}
```
阅读全文
相关推荐
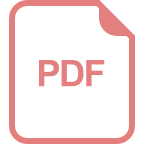
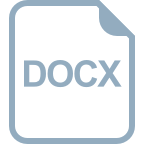
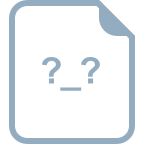









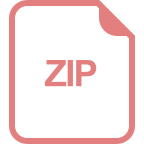
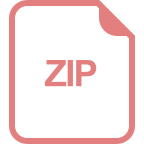
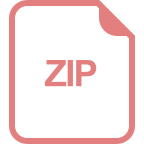
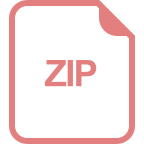