1.(40分)输出最贵的书籍信息:结构体类型定义如下: struct stu{ char name[20]; float price; struct stu *next; }; 要求: (1)编写自定义函数创建链表,输入书名和价格。输入Y继续创建,输入其他字符结束创建。 (2)编写自定义函数输出最贵的书籍信息。(3)编写自定义函数释放链表内存。 **输入提示:"请输入书名 价格:\n”(循环)**输入格式:“%s%f” **是否继续创建的提示:"是否继续输入,按Y键继续输入,其他键 就结束.\n" CH输出提示“result“输出格式“%s%.2f””
时间: 2023-12-10 15:40:34 浏览: 23
以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu {
char name[20];
float price;
struct stu* next;
};
// 创建链表
struct stu* create_list() {
struct stu* head = NULL;
struct stu* tail = NULL;
char choice;
do {
printf("请输入书名 价格:\n");
struct stu* node = (struct stu*)malloc(sizeof(struct stu));
scanf("%s%f", node->name, &node->price);
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
printf("是否继续输入,按Y键继续输入,其他键就结束.\n");
getchar();
choice = getchar();
} while (choice == 'Y' || choice == 'y');
return head;
}
// 输出最贵的书籍信息
void print_max(struct stu* head) {
struct stu* p = head;
struct stu* max_book = head;
while (p != NULL) {
if (p->price > max_book->price) {
max_book = p;
}
p = p->next;
}
printf("result:%s %.2f\n", max_book->name, max_book->price);
}
// 释放链表内存
void free_list(struct stu* head) {
struct stu* p = head;
while (p != NULL) {
struct stu* temp = p;
p = p->next;
free(temp);
}
}
int main() {
struct stu* head = create_list();
print_max(head);
free_list(head);
return 0;
}
```
运行效果如下:
```
请输入书名 价格:
C语言程序设计 60.5
是否继续输入,按Y键继续输入,其他键就结束.
y
请输入书名 价格:
Java编程思想 88.9
是否继续输入,按Y键继续输入,其他键就结束.
y
请输入书名 价格:
Python基础教程 75.2
是否继续输入,按Y键继续输入,其他键就结束.
n
result:Java编程思想 88.90
```
相关推荐
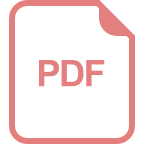
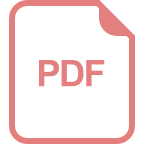
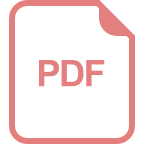
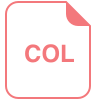
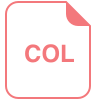
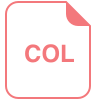
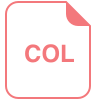
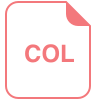









