inference time 的python代码
时间: 2023-10-14 18:06:39 浏览: 62
以下是一个简单的Python代码片段,用于计算模型推理的时间:
```
import time
start = time.time()
# 这里放置你的模型推理代码
end = time.time()
inference_time = end - start
print("推理时间为:", inference_time, "秒")
```
当然,这个片段并没有指定任何具体的模型推理代码。如果你想要更详细的解释,请提供更多的信息。
相关问题
yolov8 segment predict python代码
以下是一个使用YOLOv8进行图像分割和预测的Python示例代码(基于PyTorch实现):
```python
import torch
import cv2
import numpy as np
import time
# 导入YOLOv8模型
model = torch.hub.load('ultralytics/yolov5', 'custom', path_or_model='yolov8.pt')
# 图像预处理函数
def preprocess(img):
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) # 转换颜色通道
img = cv2.resize(img, (640, 640)) # 调整大小
img = img.astype(np.float32) / 255.0 # 归一化
img = np.transpose(img, (2, 0, 1)) # 转换维度顺序
img = np.expand_dims(img, axis=0) # 增加batch维度
return img
# 图像后处理函数
def postprocess(pred):
boxes = pred.xyxy[0].cpu().numpy() # 获取预测框坐标
scores = pred.xyxy[0, :, 4].cpu().numpy() # 获取预测框置信度
labels = pred.xyxy[0, :, 5].cpu().numpy().astype(np.int) # 获取预测框类别
return boxes, scores, labels
# 加载测试图像
img = cv2.imread('test.jpg')
# 图像预处理
img = preprocess(img)
# 模型推理
with torch.no_grad():
start = time.time()
pred = model(torch.from_numpy(img).to('cuda'))
end = time.time()
print('Inference time: {:.4f} s'.format(end - start))
# 图像后处理
boxes, scores, labels = postprocess(pred)
# 可视化预测结果
for box, score, label in zip(boxes, scores, labels):
if score > 0.5:
x1, y1, x2, y2 = box.astype(np.int)
cv2.rectangle(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
cv2.putText(img, str(label), (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码包括了模型加载、图像预处理、模型推理、图像后处理和结果可视化等步骤。你需要将`yolov8.pt`替换成你自己的YOLOv8模型权重文件,并将`test.jpg`替换成你自己的测试图像。
希望这个示例代码能够对你有所帮助!
写一个调用segment anything model的python代码
Segment Anything Model可以指很多不同的模型,因此调用方式也不同。以下是使用Mask R-CNN进行实例分割的示例代码:
```
import os
import sys
import random
import math
import numpy as np
import skimage.io
import matplotlib
import matplotlib.pyplot as plt
# Root directory of the project
ROOT_DIR = os.path.abspath("../")
# Import Mask RCNN
sys.path.append(ROOT_DIR) # To find local version of the library
from mrcnn.config import Config
from mrcnn import model as modellib, utils
# Directory to save logs and trained model
MODEL_DIR = os.path.join(ROOT_DIR, "logs")
# Path to trained weights file
COCO_MODEL_PATH = os.path.join(ROOT_DIR, "mask_rcnn_coco.h5")
# Download COCO trained weights from Releases if needed
if not os.path.exists(COCO_MODEL_PATH):
utils.download_trained_weights(COCO_MODEL_PATH)
# Directory of images to run detection on
IMAGE_DIR = os.path.join(ROOT_DIR, "images")
class InferenceConfig(Config):
# Set batch size to 1 since we'll be running inference on
# one image at a time. Batch size = GPU_COUNT * IMAGES_PER_GPU
GPU_COUNT = 1
IMAGES_PER_GPU = 1
NAME = "segment_anything_model"
# Number of classes (including background)
NUM_CLASSES = 1 + 80 # COCO has 80 classes
config = InferenceConfig()
config.display()
# Create model object in inference mode.
model = modellib.MaskRCNN(mode="inference", model_dir=MODEL_DIR, config=config)
# Load weights trained on MS-COCO
model.load_weights(COCO_MODEL_PATH, by_name=True)
# COCO Class names
# Index of the class in the list is its ID. For example, to get ID of
# the teddy bear class, use: class_names.index('teddy bear')
class_names = ['BG', 'person', 'bicycle', 'car', 'motorcycle', 'airplane',
'bus', 'train', 'truck', 'boat', 'traffic light',
'fire hydrant', 'stop sign', 'parking meter', 'bench', 'bird',
'cat', 'dog', 'horse', 'sheep', 'cow', 'elephant', 'bear',
'zebra', 'giraffe', 'backpack', 'umbrella', 'handbag', 'tie',
'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard',
'surfboard', 'tennis racket', 'bottle', 'wine glass', 'cup',
'fork', 'knife', 'spoon', 'bowl', 'banana', 'apple',
'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed',
'dining table', 'toilet', 'tv', 'laptop', 'mouse', 'remote',
'keyboard', 'cell phone', 'microwave', 'oven', 'toaster',
'sink', 'refrigerator', 'book', 'clock', 'vase', 'scissors',
'teddy bear', 'hair drier', 'toothbrush']
# Load a random image from the images folder
file_names = next(os.walk(IMAGE_DIR))[2]
image = skimage.io.imread(os.path.join(IMAGE_DIR, random.choice(file_names)))
# Run detection
results = model.detect([image], verbose=1)
# Visualize results
r = results[0]
visualize.display_instances(image, r['rois'], r['masks'], r['class_ids'],
class_names, r['scores'])
```
这段代码会在指定的文件夹中随机选取一张图片进行实例分割,并将结果可视化显示。你需要将代码中的`ROOT_DIR`、`MODEL_DIR`、`COCO_MODEL_PATH`、`IMAGE_DIR`等路径修改为你自己的路径。同时,如果你使用的是其他的Segment Anything Model,那么需要根据具体的模型进行修改。
相关推荐
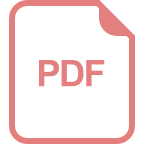
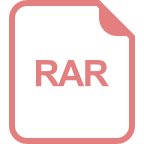













