public class Date { private int year, month, day; public void Date(int y,int m,int d) { //定义有参构造方法 year=y; month=m; day=d; } public Date(){ //定义无参构造方法 Date(2018,6,15); } public void setMonth(int month) {//给成员变量month赋值 month=month; } public int getMonth(){ returm month; } public void print(){ System.out.println("date is "+ this.year+"-"+this.month+"-"+this.day); } public void main(String args[]){ Date oday = new Date(2018,7); print(); } } 找出7处错误
时间: 2024-02-24 15:55:27 浏览: 28
1. 构造方法的名称应该与类名相同,因此应该将 `void Date` 改为 `public Date`。
2. 在无参构造方法中,应该使用 `this` 关键字调用有参构造方法,因此应该将 `Date(2018,6,15)` 改为 `this(2018,6,15)`。
3. 在 `setMonth` 方法中,应该使用 `this` 关键字指定赋值的对象,因此应该将 `month=month` 改为 `this.month=month`。
4. 在 `getMonth` 方法中,应该将 `returm` 改为 `return`。
5. 在 `print` 方法中,应该将 `this.day` 改为 `day`。
6. `main` 方法应该是静态的,因此应该将 `public void main(String args[])` 改为 `public static void main(String args[])`。
7. 在 `main` 方法中,应该使用 `oday` 对象调用 `print` 方法,因此应该将 `print()` 改为 `oday.print()`。
修改后的代码如下:
```
public class Date {
private int year, month, day;
public Date(int y, int m, int d) { // 定义有参构造方法
year = y;
month = m;
day = d;
}
public Date() { // 定义无参构造方法
this(2018, 6, 15);
}
public void setMonth(int month) { // 给成员变量month赋值
this.month = month;
}
public int getMonth() {
return month;
}
public void print() {
System.out.println("date is " + this.year + "-" + this.month + "-" + this.day);
}
public static void main(String args[]) {
Date oday = new Date(2018, 7, 1);
oday.print();
}
}
```
相关推荐
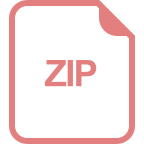
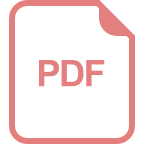
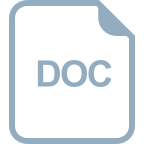














