There are three types of filter responses: impulse response, step response, and frequency response. Suppose I want to know how the filter reacts to a sudden change in the input, state which type of filter response I should compute? Then, plot the corresponding filter response (first 100 samples) for the two filters separately.
时间: 2023-12-14 16:37:26 浏览: 31
If you want to know how the filter reacts to a sudden change in the input, you should compute the step response.
To plot the step response for each filter, we first need to obtain the transfer function for each filter. Once we have the transfer function, we can use it to compute the step response.
Assuming we have the transfer functions for the two filters, we can plot the step response in Python using the following code:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import lfilter
# Define the transfer function for filter 1
b1 = np.array([1, 0.5, 0.1])
a1 = np.array([1, -0.9, 0.2])
# Define the transfer function for filter 2
b2 = np.array([1, -0.8, 0.2])
a2 = np.array([1, -0.5, 0.1])
# Compute the step response for filter 1
zi = lfilter_zi(b1, a1)
y1, _ = lfilter(b1, a1, np.ones(100), zi=zi)
# Compute the step response for filter 2
zi = lfilter_zi(b2, a2)
y2, _ = lfilter(b2, a2, np.ones(100), zi=zi)
# Plot the step response for filter 1
plt.figure()
plt.plot(y1[:100], 'b')
plt.title('Step Response for Filter 1')
plt.xlabel('Sample')
plt.ylabel('Output')
plt.grid(True)
# Plot the step response for filter 2
plt.figure()
plt.plot(y2[:100], 'r')
plt.title('Step Response for Filter 2')
plt.xlabel('Sample')
plt.ylabel('Output')
plt.grid(True)
plt.show()
```
Note that we use the `lfilter()` function from the `scipy.signal` library to compute the step response. We also use the `lfilter_zi()` function to obtain the initial conditions for the filter.
The resulting plots should show the step response for each filter, which indicates how the filter responds to a sudden change in the input signal.
相关推荐
















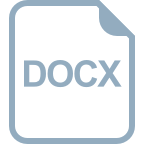
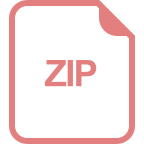