#include <stdio.h> #include <stdlib.h> #define STACK_SIZE 100 typedef struct { int top; int data[STACK_SIZE]; } Stack; void initStack(Stack* s) { s->top = -1; } int isStackEmpty(Stack* s) { return s->top == -1; } int isStackFull(Stack* s) { return s->top == STACK_SIZE - 1; } void push(Stack* s, int x) { if (isStackFull(s)) { printf("Stack is full\n"); exit(1); } s->data[++s->top] = x; } int pop(Stack* s) { if (isStackEmpty(s)) { printf("Stack is empty\n"); exit(1); } return s->data[s->top--]; } int main() { int n; Stack s; initStack(&s); printf("输入一个十进制数: "); scanf_s("%d", &n); while (n > 0) { push(&s, n % 2); n /= 2; } printf("二进制为: "); while (!isStackEmpty(&s)) { printf("%d", pop(&s)); } printf("\n"); return 0; }该实验收获
时间: 2024-03-04 16:53:32 浏览: 148
这个实验让我们学习了如何使用栈来进行进制转换,同时也熟悉了栈的基本操作。
具体来说,我们学会了如何定义一个栈的结构体,并通过指针来进行栈的操作。我们也学会了如何判断栈是否为空或已满,以及如何进行入栈和出栈操作。
通过本实验,我们还学习了如何使用while循环来进行重复操作,让程序可以反复执行某些操作直到满足结束条件。同时,我们也学会了如何使用scanf_s函数来进行输入,以及如何使用printf函数来进行输出。
通过这个实验,我们不仅掌握了栈的基本操作,还提高了编程能力,学会了如何将一个实际问题抽象成程序,并通过代码来解决问题。
相关问题
本题目要求利用栈的基本操作,实现一个清空顺序栈S的算法。 #include <stdio.h> #include <math.h> #include <stdlib.h> typedef int DataType; /*栈中允许存储的元素的最大个数*/ #define STACKSIZE 100 /* 顺序栈的定义 */ typedef struct
{
DataType data[STACKSIZE]; // 存储栈的元素
int top; // 栈顶指针,指向栈顶元素在data数组中的下标
} SeqStack;
/* 初始化栈 */
void InitStack(SeqStack *S)
{
S->top = -1; // 栈顶指针初始化为-1,表示栈为空
}
/* 判断栈是否为空 */
int StackEmpty(SeqStack S)
{
return (S.top == -1);
}
/* 判断栈是否已满 */
int StackFull(SeqStack S)
{
return (S.top == STACKSIZE - 1);
}
/* 进栈 */
int Push(SeqStack *S, DataType x)
{
if (StackFull(*S)) // 栈已满,不能再进栈
{
return 0;
}
else
{
S->top++; // 栈顶指针加1
S->data[S->top] = x; // 将x存入栈顶元素
return 1;
}
}
/* 出栈 */
int Pop(SeqStack *S, DataType *x)
{
if (StackEmpty(*S)) // 栈为空,不能出栈
{
return 0;
}
else
{
*x = S->data[S->top]; // 将栈顶元素出栈
S->top--; // 栈顶指针减1
return 1;
}
}
/* 清空栈 */
void ClearStack(SeqStack *S)
{
S->top = -1; // 直接将栈顶指针置为-1,表示栈为空
}
#include <stdio.h> #include <stdlib.h> #include <ctype.h> #include <math.h> #define TRUE 1 #define FALSE 0 // 表达式树结点结构体 typedef struct _etNode{ char data; // 结点数据:操作符或操作数 struct _etNode* left; // 左子树指针 struct _etNode* right; // 右子树指针 }ET
Node;
// 定义栈结构体
typedef struct _stack{
int top; // 栈顶指针
int size; // 栈的大小
ETNode** data; // 栈的数据
}Stack;
// 创建栈
Stack* createStack(int size){
Stack* s = (Stack*)malloc(sizeof(Stack));
s->top = -1;
s->size = size;
s->data = (ETNode**)malloc(sizeof(ETNode*)*size);
return s;
}
// 判断栈为空
int isEmptyStack(Stack* s){
return s->top == -1;
}
// 判断栈是否已满
int isFullStack(Stack* s){
return s->top == s->size-1;
}
// 入栈
void pushStack(Stack* s, ETNode* e){
if(isFullStack(s)){
printf("Stack is full!\n");
return;
}
s->data[++s->top] = e;
}
// 出栈
ETNode* popStack(Stack* s){
if(isEmptyStack(s)){
printf("Stack is empty!\n");
return NULL;
}
return s->data[s->top--];
}
// 获取栈顶元素
ETNode* getTopStack(Stack* s){
if(isEmptyStack(s)){
printf("Stack is empty!\n");
return NULL;
}
return s->data[s->top];
}
// 判断字符是否为操作符
int isOperator(char c){
switch(c){
case '+':
case '-':
case '*':
case '/':
case '^':
return TRUE;
default:
return FALSE;
}
}
// 创建表达式树
ETNode* createET(char* expr){
Stack* s = createStack(strlen(expr));
int i;
for(i=0; expr[i]!='\0'; i++){
if(isdigit(expr[i])){
// 如果是数字,则创建一个叶子结点
ETNode* node = (ETNode*)malloc(sizeof(ETNode));
node->data = expr[i];
node->left = NULL;
node->right = NULL;
pushStack(s, node);
}
else if(isOperator(expr[i])){
// 如果是操作符,则创建一个新的结点,并将栈顶的两个结点作为它的左右孩子
ETNode* node = (ETNode*)malloc(sizeof(ETNode));
node->data = expr[i];
node->right = popStack(s);
node->left = popStack(s);
pushStack(s, node);
}
else{
// 如果是无效字符,则忽略
continue;
}
}
return popStack(s);
}
// 计算表达式树的值
double calculateET(ETNode* root){
if(root == NULL){
return 0;
}
if(root->left == NULL && root->right == NULL){
// 如果是叶子结点,则返回它的值
return atof(&root->data);
}
double leftValue = calculateET(root->left);
double rightValue = calculateET(root->right);
switch(root->data){
case '+':
return leftValue + rightValue;
case '-':
return leftValue - rightValue;
case '*':
return leftValue * rightValue;
case '/':
return leftValue / rightValue;
case '^':
return pow(leftValue, rightValue);
default:
return 0;
}
}
int main(){
char expr[100];
printf("请输入中缀表达式:");
gets(expr);
ETNode* root = createET(expr);
printf("表达式树创建成功!\n");
printf("表达式的计算结果为:%lf\n", calculateET(root));
return 0;
}
阅读全文
相关推荐
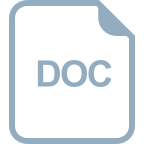
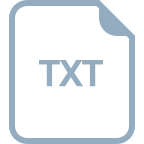
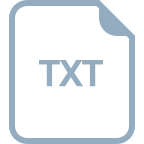














