#include <stdio.h> #include <stdlib.h> #define Stack_Size 50 typedef struct { int data[Stack_Size]; int top; }SqStack; bool InitStack(SqStack &s) { s.top=-1; return true; } bool isEmptyStack(SqStack s) { if(s.top==-1) return true; else return false; } bool isFullStack(SqStack s) { if(s.top==Stack_Size-1) return true; else return false; } bool Push(SqStack &s,int x) { if(isFullStack(s)==1) return false; else { s.top++; s.data[s.top]=x; return true; } } bool Pop(SqStack &s,int &x) { if(isEmptyStack(s)==1) return false; else { x=s.data[s.top]; s.top--; return true; } } int main() { SqStack s; InitStack(s); int x,y; printf("请输入需要进栈的元素(999结束):\n"); scanf("%d",&x); while(x!=999) { Push(s,x); scanf("%d",&x); } if(isEmptyStack(s)==1) printf("该栈为空\n"); else printf("该栈不为空\n"); if(isFullStack(s)==1) printf("该栈已满\n"); else printf("该栈未满\n"); Pop(s,x); printf("出栈的元素为:%d\n",x); printf("此时栈中的元素为:\n"); } 怎么改正
时间: 2024-01-07 19:05:17 浏览: 66
代码存在几个问题:
1. 栈的数据类型定义应该加上typedef,否则在主函数中定义栈变量时需要写成struct SqStack s;。
2. Pop函数中没有判断栈是否为空,如果栈为空,就不能进行出栈操作。
3. 主函数中没有输出栈中的元素。
下面是修改后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define Stack_Size 50
typedef struct {
int data[Stack_Size];
int top;
} SqStack;
typedef enum {
false, true
} bool;
bool InitStack(SqStack &s) {
s.top = -1;
return true;
}
bool isEmptyStack(SqStack s) {
if (s.top == -1)
return true;
else
return false;
}
bool isFullStack(SqStack s) {
if (s.top == Stack_Size - 1)
return true;
else
return false;
}
bool Push(SqStack &s, int x) {
if (isFullStack(s) == 1)
return false;
else {
s.top++;
s.data[s.top] = x;
return true;
}
}
bool Pop(SqStack &s, int &x) {
if (isEmptyStack(s) == 1)
return false;
else {
x = s.data[s.top];
s.top--;
return true;
}
}
int main() {
SqStack s;
InitStack(s);
int x, y;
printf("请输入需要进栈的元素(999结束):\n");
scanf("%d", &x);
while (x != 999) {
Push(s, x);
scanf("%d", &x);
}
if (isEmptyStack(s) == 1)
printf("该栈为空\n");
else
printf("该栈不为空\n");
if (isFullStack(s) == 1)
printf("该栈已满\n");
else
printf("该栈未满\n");
if (Pop(s, x) == true) {
printf("出栈的元素为:%d\n", x);
} else {
printf("栈为空,无法进行出栈操作!\n");
}
printf("此时栈中的元素为:\n");
while (isEmptyStack(s) == false) {
Pop(s, x);
printf("%d\n", x);
}
return 0;
}
```
阅读全文
相关推荐
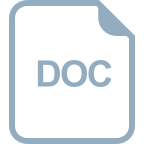
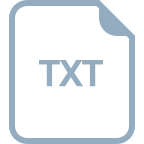
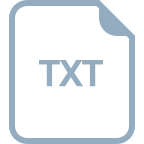
















