C语言创建一个单链表,有输入,查询,取值,插入,删除,输出的功能
时间: 2024-05-30 16:16:02 浏览: 19
#include<stdio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
void insert(int x){
struct node *temp=(struct node*)malloc(sizeof(struct node));
temp->data=x;
temp->next=NULL;
if(head==NULL){
head=temp;
}
else{
struct node *temp1=head;
while(temp1->next!=NULL){
temp1=temp1->next;
}
temp1->next=temp;
}
}
void delete(int x){
struct node *temp1=head;
if(x==1){
head=temp1->next;
free(temp1);
return;
}
int i;
for(i=0;i<x-2;i++){
temp1=temp1->next;
}
struct node *temp2=temp1->next;
temp1->next=temp2->next;
free(temp2);
}
int retrieve(int x){
struct node *temp=head;
int i;
for(i=0;i<x-1;i++){
temp=temp->next;
}
return temp->data;
}
void search(int x){
struct node *temp=head;
int i,flag=0;
for(i=0;i<x-1;i++){
temp=temp->next;
}
printf("The value at position %d is: %d\n",x,temp->data);
}
void display(){
struct node *temp=head;
while(temp!=NULL){
printf("%d ",temp->data);
temp=temp->next;
}
printf("\n");
}
int main(){
int ch=0,x,pos;
while(ch!=6){
printf("Enter the choice:\n");
printf("1. Insert an element in the list\n");
printf("2. Delete an element from the list\n");
printf("3. Retrieve an element from the list\n");
printf("4. Search for an element in the list\n");
printf("5. Display the list\n");
printf("6. Exit\n");
scanf("%d",&ch);
switch(ch){
case 1: printf("Enter the element to be inserted: ");
scanf("%d",&x);
insert(x);
break;
case 2: printf("Enter the position of the element to be deleted: ");
scanf("%d",&pos);
delete(pos);
break;
case 3: printf("Enter the position of the element to be retrieved: ");
scanf("%d",&pos);
x=retrieve(pos);
printf("The value at position %d is: %d\n",pos,x);
break;
case 4: printf("Enter the position of the element to be searched: ");
scanf("%d",&pos);
search(pos);
break;
case 5: display();
break;
case 6: printf("Exiting...\n");
break;
default: printf("Invalid choice\n");
}
}
return 0;
}
相关推荐
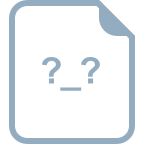
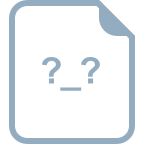










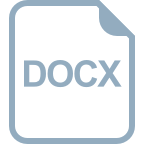