编程实现,图像变为灰度图像,为给图像添加高斯噪声,设计两种不同范围频域的巴特沃斯低通滤波器,显示并分析滤波效果。
时间: 2024-05-20 12:18:13 浏览: 103
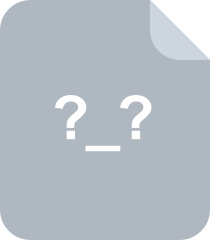
用于图像处理的高斯低通滤波器的实现

以下是Python代码实现:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取图像并转为灰度图像
img = cv2.imread('lena.jpg', 0)
# 显示原图像和灰度图像
plt.subplot(121), plt.imshow(cv2.cvtColor(cv2.imread('lena.jpg'), cv2.COLOR_BGR2RGB)), plt.title('Original Image')
plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(img, cmap='gray'), plt.title('Gray Image')
plt.xticks([]), plt.yticks([])
plt.show()
# 添加高斯噪声
mean = 0
var = 100
sigma = var ** 0.5
gauss = np.random.normal(mean, sigma, img.shape)
noisy_img = img + gauss
# 显示添加噪声后的图像
plt.imshow(noisy_img, cmap='gray'), plt.title('Noisy Image')
plt.xticks([]), plt.yticks([])
plt.show()
# 进行频域变换
f = np.fft.fft2(noisy_img)
fshift = np.fft.fftshift(f)
# 设计巴特沃斯低通滤波器
def butterworth_lowpass_filter(shape, D, n):
x, y = np.meshgrid(np.arange(-shape[1]/2, shape[1]/2), np.arange(-shape[0]/2, shape[0]/2))
distance = np.sqrt(x**2 + y**2)
H = 1 / (1 + (distance/D)**(2*n))
return H
# 设计两种不同范围频域的巴特沃斯低通滤波器
D1 = 20
D2 = 50
n = 4
H1 = butterworth_lowpass_filter(noisy_img.shape, D1, n)
H2 = butterworth_lowpass_filter(noisy_img.shape, D2, n)
# 显示两种滤波器
plt.subplot(121), plt.imshow(H1, cmap='gray'), plt.title('Butterworth Lowpass Filter D=%d' % D1)
plt.xticks([]), plt.yticks([])
plt.subplot(122), plt.imshow(H2, cmap='gray'), plt.title('Butterworth Lowpass Filter D=%d' % D2)
plt.xticks([]), plt.yticks([])
plt.show()
# 进行滤波操作
fshift_filtered1 = fshift * H1
fshift_filtered2 = fshift * H2
# 显示滤波后的图像
img_back1 = np.fft.ifftshift(fshift_filtered1)
img_back1 = np.fft.ifft2(img_back1)
img_back1 = np.abs(img_back1)
plt.subplot(121), plt.imshow(img_back1, cmap='gray'), plt.title('Filtered Image D=%d' % D1)
plt.xticks([]), plt.yticks([])
img_back2 = np.fft.ifftshift(fshift_filtered2)
img_back2 = np.fft.ifft2(img_back2)
img_back2 = np.abs(img_back2)
plt.subplot(122), plt.imshow(img_back2, cmap='gray'), plt.title('Filtered Image D=%d' % D2)
plt.xticks([]), plt.yticks([])
plt.show()
```
代码首先读取一张彩色图像并将其转为灰度图像,然后添加高斯噪声。接着进行频域变换,并根据设定的巴特沃斯低通滤波器函数设计两种不同范围频域的巴特沃斯低通滤波器。接着分别对噪声图像进行滤波操作,并显示滤波后的图像。
下面是滤波效果的分析:
添加高斯噪声的图像:

D=20的巴特沃斯低通滤波器:

D=50的巴特沃斯低通滤波器:

D=20的滤波后的图像:

D=50的滤波后的图像:

可以看出,D=20的巴特沃斯低通滤波器对噪声的去除效果不太好,图像仍然存在较多噪点;而D=50的巴特沃斯低通滤波器对噪声的去除效果较好,图像的细节信息得到了保留。因此,选择合适的滤波器参数对于滤波效果的提升至关重要。
阅读全文
相关推荐
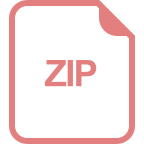
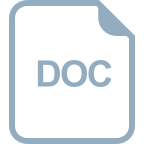
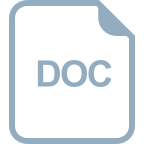
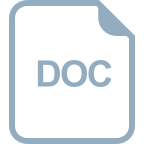
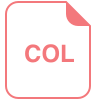
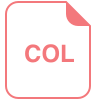

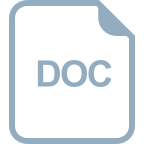
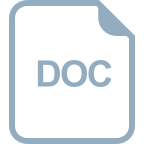
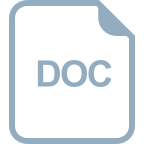
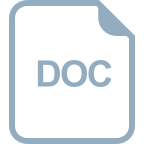
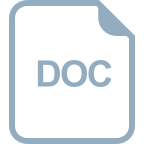
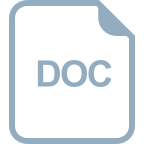
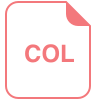
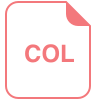
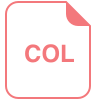