二叉树搜索,python代码
时间: 2023-08-31 08:32:08 浏览: 50
### 回答1:
二叉树搜索是在二叉搜索树中查找特定值的过程。二叉搜索树是一种特殊的二叉树,其中每个节点的值都大于其左子树中的所有节点的值,而小于其右子树中的所有节点的值。
下面是用 Python 实现二叉树搜索的示例代码:
```
class Node:
def __init__(self, value):
self.left = None
self.right = None
self.value = value
def search(root, value):
if root is None or root.value == value:
return root
if value < root.value:
return search(root.left, value)
else:
return search(root.right, value)
# 建立二叉搜索树
root = Node(8)
root.left = Node(3)
root.right = Node(10)
root.left.left = Node(1)
root.left.right = Node(6)
root.right.right = Node(14)
# 查找值为 6 的节点
result = search(root, 6)
if result:
print("Found node with value 6")
else:
print("Node with value 6 not found")
```
在这段代码中,我们首先建立了一棵二叉搜索树,然后使用递归函数 `search` 来查找值为 6 的节点。如果找到了该节点,就会打印 "Found node with value 6";如果没有找到,就会打印 "Node with value 6 not found"。
### 回答2:
二叉树搜索是指在二叉树中查找特定值的过程。下面是用Python编写的二叉树搜索代码示例:
```python
# 定义二叉树节点类
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
# 二叉树搜索函数
def binary_search(root, target):
if not root or root.value == target:
return root
if root.value < target:
return binary_search(root.right, target)
return binary_search(root.left, target)
# 创建二叉树
def build_binary_tree():
root = Node(5)
root.left = Node(3)
root.right = Node(7)
root.left.left = Node(2)
root.left.right = Node(4)
root.right.left = Node(6)
root.right.right = Node(8)
return root
# 测试二叉树搜索
if __name__ == "__main__":
root = build_binary_tree()
target = 4
result = binary_search(root, target)
if result:
print("找到了目标值", result.value)
else:
print("未找到目标值")
```
以上代码中,首先定义一个节点类,包含节点的值以及左右子节点。然后定义了二叉树搜索函数`binary_search`,该函数会在给定的二叉树中查找目标值。如果找到目标值,则返回匹配的节点;若未找到,则返回`None`。最后通过调用`build_binary_tree`函数创建一个二叉树,并测试二叉树搜索函数。如果找到目标值,则打印"找到了目标值"以及对应的节点值;若未找到目标值,则打印"未找到目标值"。
### 回答3:
二叉树搜索(binary search tree)是一种常用的数据结构,它具有以下特点:对于任意节点,它的左子树中的所有节点的值都小于该节点的值,而右子树中的所有节点的值都大于该节点的值。搜索操作即在二叉树中查找指定的值。
下面是用python编写的二叉树搜索的代码示例:
```python
# 定义二叉树节点类
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
# 插入节点的函数
def insert(root, value):
if root is None:
root = Node(value)
else:
if value < root.value:
if root.left is None:
root.left = Node(value)
else:
insert(root.left, value)
else:
if root.right is None:
root.right = Node(value)
else:
insert(root.right, value)
# 搜索函数
def search(root, value):
if root is None or root.value == value:
return root
if value < root.value:
return search(root.left, value)
else:
return search(root.right, value)
# 创建二叉树
root = Node(5)
insert(root, 3)
insert(root, 7)
insert(root, 2)
insert(root, 4)
insert(root, 6)
insert(root, 8)
# 在二叉树中搜索指定值
value_to_search = 4
result = search(root, value_to_search)
if result is None:
print(f"在二叉树中未找到值为{value_to_search}的节点")
else:
print(f"在二叉树中找到了值为{value_to_search}的节点")
```
以上代码中包含了二叉树的插入和搜索函数。首先创建了一个具有七个节点的二叉树,并在其中搜索值为4的节点。最后根据搜索结果输出相应的信息。
希望以上代码能够对你有所帮助。
相关推荐
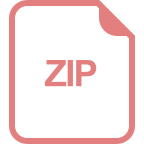














