python求二叉树的宽度
时间: 2023-11-29 11:00:46 浏览: 40
计算二叉树的宽度需要对每一层进行遍历,统计每一层的节点数,并取最大值作为二叉树的宽度。可以使用 BFS(广度优先搜索)算法来实现。
下面是一个示例代码:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def widthOfBinaryTree(root: TreeNode) -> int:
if not root:
return 0
queue = [(root, 0)]
max_width = 0
while queue:
level_length = len(queue)
_, level_start = queue[0]
for i in range(level_length):
node, col_index = queue.pop(0)
if node.left:
queue.append((node.left, 2 * col_index))
if node.right:
queue.append((node.right, 2 * col_index + 1))
max_width = max(max_width, col_index - level_start + 1)
return max_width
```
其中,```queue``` 存储了每个节点的列索引和所在层数,```level_length``` 记录当前层节点数,```level_start``` 记录当前层的起始列索引,```col_index``` 记录当前节点的列索引。
在每一层遍历结束后,计算当前层的宽度并更新最大宽度 ```max_width```。最后返回最大宽度即可。
相关推荐
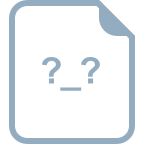














