Python二叉树算法实战:构建与遍历的多样化方法
发布时间: 2024-09-09 20:38:59 阅读量: 71 订阅数: 48 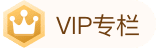
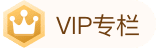
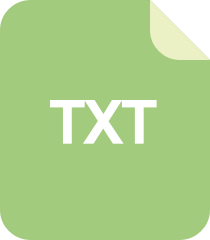
数据结构与算法:二叉树层次遍历及其应用

# 1. 二叉树算法的理论基础
在计算机科学中,二叉树是一种重要的数据结构,它具有独特的层次性和分枝性,使得数据的存储和管理变得更加高效。二叉树算法在各种搜索、排序、树形数据结构的设计等领域拥有广泛的应用。本章将深入探讨二叉树的基础概念,包括其定义、性质、类型等理论知识,为深入学习二叉树算法打下坚实的基础。
## 1.1 二叉树的基本概念
二叉树是每个节点最多有两个子树的树结构,通常子树被称作“左子树”和“右子树”。节点的度为该节点拥有的子树数目。在二叉树中,有一个根节点,根节点下方是层级结构,从根节点开始,每一层节点都是下一层节点的父节点。
```plaintext
A
/ \
B C
/ \ / \
D E F G
```
例如,上述的树结构中,节点B是节点D和E的父亲,节点A是根节点。
## 1.2 二叉树的性质
二叉树有若干重要的性质,对于理解二叉树的结构和算法至关重要。例如:
- 第i层最多有2^(i-1)个节点(i≥1)
- 深度为k的二叉树最多有2^k - 1个节点(k≥1)
- 二叉树的度为0的节点(叶子节点)总是比度为2的节点多一个
## 1.3 二叉树的遍历
遍历二叉树是访问树中每个节点一次且仅一次的过程。常见的遍历方法包括前序遍历、中序遍历和后序遍历。每种遍历方法都有其特定的访问顺序,这在后续的章节中将有更详细的讲解。
例如:
- 前序遍历:根节点 -> 左子树 -> 右子树
- 中序遍历:左子树 -> 根节点 -> 右子树
- 后序遍历:左子树 -> 右子树 -> 根节点
理解二叉树的基础理论是掌握其算法实现的第一步,本章内容仅提供一个概览,下一章我们将深入到二叉树的构建方法,进一步理解并实现二叉树结构。
# 2. 二叉树的构建方法
### 2.1 基本构建方法
#### 2.1.1 递归构建法
递归构建法是二叉树构建中最直观的方法之一。它利用了二叉树的递归性质,即每个节点都是其子树的根节点。在实现上,通常会先构造根节点,然后递归地构造左右子树。以下是使用Python实现的递归构建二叉树的示例代码:
```python
class TreeNode:
def __init__(self, value):
self.val = value
self.left = None
self.right = None
def insert_node(root, value):
if root is None:
return TreeNode(value)
if value < root.val:
root.left = insert_node(root.left, value)
else:
root.right = insert_node(root.right, value)
return root
```
这段代码中,`insert_node` 函数以递归的方式插入新的节点值 `value` 到二叉搜索树中。函数首先检查当前节点是否为空,如果是,就创建一个新的 `TreeNode` 实例并返回。如果当前节点不为空,它将比较 `value` 与当前节点的值,然后递归地在左子树或右子树中插入新的节点。
递归构建法的优点在于代码简洁易懂,但缺点是可能会因为递归调用导致栈溢出,特别是当树的深度过大时。
#### 2.1.2 迭代构建法
为了克服递归构建法中的栈溢出问题,我们可以采用迭代的方式构建二叉树。迭代构建法使用一个队列来存储节点,通过循环和队列的先进先出(FIFO)特性来构建树结构。以下是使用Python实现的迭代构建二叉树的示例代码:
```python
from collections import deque
def build_tree_nodes(values):
if not values:
return None
root = TreeNode(values[0])
queue = deque([root])
i = 1
while queue and i < len(values):
node = queue.popleft()
if node:
left_val = values[i]
i += 1
if left_val is not None:
node.left = TreeNode(left_val)
queue.append(node.left)
if i < len(values):
right_val = values[i]
i += 1
if right_val is not None:
node.right = TreeNode(right_val)
queue.append(node.right)
return root
```
在这段代码中,`build_tree_nodes` 函数接收一个列表 `values`,其中的值按照二叉树的层次遍历的顺序排列。函数首先创建根节点,然后初始化一个队列。接下来,通过循环和队列操作,按照层次顺序构建整棵树。
迭代构建法避免了递归带来的栈溢出问题,适用于构建任意形状的二叉树,包括完全二叉树和非完全二叉树。
### 2.2 特殊二叉树的构建
#### 2.2.1 完全二叉树与满二叉树的构造
完全二叉树和满二叉树具有特定的形状,它们在计算机科学和工程中有广泛的应用。完全二叉树是除了最后一层外,每一层都被完全填满的二叉树,且最后一层所有节点都尽可能地向左。满二叉树则是每一层都被完全填满的二叉树。
构建这两种特殊二叉树的方法主要有两种:
- 直接基于二叉树的定义进行构建。
- 通过已有的二叉树进行调整,使其成为完全二叉树或满二叉树。
以下是一个简单的实现示例:
```python
def create_complete_binary_tree(values):
if not values:
return None
root = TreeNode(values[0])
queue = deque([root])
i = 1
while queue and i < len(values):
node = queue.popleft()
if node:
node.left = TreeNode(values[i]) if i < len(values) else None
queue.append(node.left)
i += 1
if i < len(values):
node.right = TreeNode(values[i]) if i < len(values) else None
queue.append(node.right)
i += 1
return root
```
在这个函数中,我们使用队列以层次遍历的顺序构建完全二叉树。如果队列中当前节点为空,则该位置不插入节点,以此来保证树的完全性。
#### 2.2.2 平衡二叉树(AVL树)的创建
AVL树是一种自平衡的二叉搜索树,它的每个节点的左子树和右子树的高度最多差1。当在AVL树中插入或删除节点时,可能会破坏树的平衡,此时需要通过旋转操作来重新恢复平衡。以下是使用Python实现AVL树的一个基础示例:
```python
class AVLTreeNode(TreeNode):
def __init__(self, value):
super().__init__(value)
self.height = 1
def height(node):
return node.height if node else 0
def update_height(node):
node.height = max(height(node.left), height(node.right)) + 1
def balance_factor(node):
return height(node.left) - height(node.right)
def rotate_right(y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
update_height(y)
update_height(x)
return x
def rotate_left(x):
y = x.right
T2 = y.left
y.left = x
x.right = T2
update_height(x)
update_height(y)
return y
# ... 其他平衡旋转函数和AVL树节点插入/删除操作代码 ...
```
在这段代码中,我们首先定义了 `AVLTreeNode` 类,它是 `TreeNode` 的子类,并添加了节点高度的属性。`balance_factor` 函数用于计算节点的平衡因子,而 `rotate_right` 和 `rotate_left` 函数则实现了左右旋转操作,用于在节点插入或删除后恢复树的平衡。
创建AVL树的过程涉及到在树中插入节点,并在插入后检查每个节点的
0
0
相关推荐
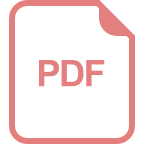
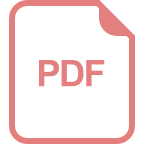
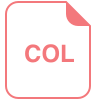
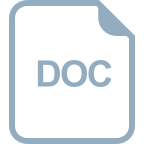
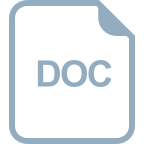
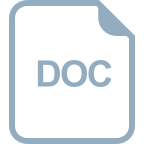
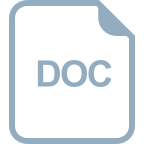