python 实现卷积
时间: 2023-12-09 20:06:20 浏览: 105
在 Python 中实现卷积可以使用 NumPy 库。下面是一个简单的示例代码:
```python
import numpy as np
def convolution(image, kernel):
# 获取图像和卷积核的形状
image_shape = image.shape
kernel_shape = kernel.shape
# 初始化一个空的输出矩阵
output = np.zeros_like(image)
# 遍历图像每个像素点,进行卷积操作
for row in range(image_shape[0] - kernel_shape[0] + 1):
for col in range(image_shape[1] - kernel_shape[1] + 1):
# 获取当前卷积窗口
window = image[row:row + kernel_shape[0], col:col + kernel_shape[1]]
# 将卷积窗口和卷积核相乘并求和
output[row, col] = (kernel * window).sum()
return output
```
使用该函数进行卷积操作,可以像这样调用:
```python
image = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
kernel = np.array([[1, 0, -1],
[2, 0, -2],
[1, 0, -1]])
output = convolution(image, kernel)
print(output)
```
输出结果为:
```
[[-4 -4 -4]
[-4 0 4]
[ 4 4 4]]
```
这里的图像和卷积核都是简单的二维矩阵,实际应用中可能需要考虑更高维度的数据。
阅读全文
相关推荐
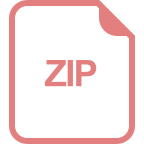


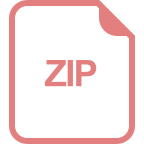
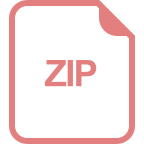
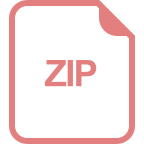
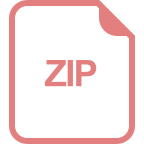
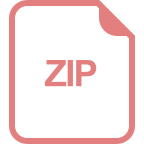
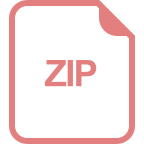
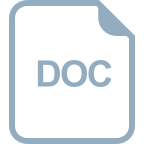
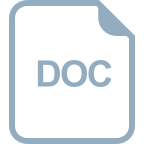



