如何在Cocos creator JS里面 写一个允许触屏是代码
时间: 2023-09-07 09:05:35 浏览: 155
### 回答1:
在 Cocos Creator 中使用 JavaScript 编写触屏事件代码的方法如下:
1. 在场景中添加一个节点,并在该节点上添加脚本组件。
2. 在脚本组件中添加如下代码,以监听触屏事件:
```js
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_START, this.onTouchStart, this);
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_MOVE, this.onTouchMove, this);
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_END, this.onTouchEnd, this);
```
3. 在脚本组件中添加 onTouchStart,onTouchMove,onTouchEnd 函数来处理触屏事件。
4. 添加相应的逻辑处理函数。
示例代码:
```js
cc.Class({
extends: cc.Component,
properties: {
// foo: {
// // ATTRIBUTES:
// default: null, // The default value will be used only when the component attaching
// // to a node for the first time
// type: cc.SpriteFrame, // optional, default is typeof default
// serializable: true, // optional, default is true
// },
// bar: {
// get () {
// return this._bar;
// },
// set (value) {
// this._bar = value;
// }
// },
},
// LIFE-CYCLE CALLBACKS:
onLoad () {
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_START, this.onTouchStart, this);
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_MOVE, this.onTouchMove, this);
cc.systemEvent.on(cc.SystemEvent.EventType.TOUCH_END, this.onTouchEnd, this);
},
onTouchStart: function (event) {
// 处理触摸开始事件
},
onTouchMove: function (event) {
// 处理触摸移动事件
},
onTouchEnd: function (event) {
// 处理触摸结束事件
},
start () {
},
// update (dt) {},
});
``
### 回答2:
在Cocos Creator中,要编写一个允许触屏的代码,可以按照以下步骤进行操作:
1. 创建一个新的JavaScript脚本文件,例如"TouchScript.js"。
2. 在脚本中定义一个新的类,例如"TouchScript",并继承自cc.Component。
3. 在类的属性中定义一个触点的回调函数,例如"onTouchStart"、"onTouchMove"、"onTouchEnd"。
4. 在onLoad函数中添加触摸监听事件,通过cc.Canvas组件的node属性获取画布节点,然后调用node.on('touchstart', this.onTouchStart, this)等方法添加触摸事件回调函数。
5. 实现每个触点回调函数,并在触摸事件发生时执行相应的代码逻辑。
6. 在需要使用触点的节点上添加TouchScript.js脚本组件。
以下是一个示例代码:
```javascript
cc.Class({
extends: cc.Component,
properties: {},
onLoad () {
let canvasNode = cc.find('Canvas');
canvasNode.on('touchstart', this.onTouchStart, this);
canvasNode.on('touchmove', this.onTouchMove, this);
canvasNode.on('touchend', this.onTouchEnd, this);
},
onTouchStart (event) {
let touchPos = event.getLocation();
console.log("触摸开始,触摸位置:", touchPos);
// 添加触摸开始的逻辑代码...
},
onTouchMove (event) {
let touchPos = event.getLocation();
console.log("触摸移动,触摸位置:", touchPos);
// 添加触摸移动的逻辑代码...
},
onTouchEnd (event) {
let touchPos = event.getLocation();
console.log("触摸结束,触摸位置:", touchPos);
// 添加触摸结束的逻辑代码...
},
});
```
在需要使用触点的节点上添加TouchScript组件后,脚本中的触点回调函数就会在触摸事件发生时被触发,并执行相应的代码逻辑。你可以根据具体需求,在触点回调函数中编写相应的业务逻辑。
### 回答3:
在Cocos Creator中,可以通过监听触摸事件来实现允许触屏的代码编写。以下是一个简单的示例代码:
```javascript
// 在场景或节点的脚本中初始化触摸事件监听
// 导入cc模块
const {ccclass, property} = cc._decorator;
@ccclass
export default class YourScript extends cc.Component {
onLoad() {
// 添加触摸事件监听
this.node.on(cc.Node.EventType.TOUCH_START, this.onTouchStart, this);
this.node.on(cc.Node.EventType.TOUCH_MOVE, this.onTouchMove, this);
this.node.on(cc.Node.EventType.TOUCH_END, this.onTouchEnd, this);
this.node.on(cc.Node.EventType.TOUCH_CANCEL, this.onTouchCancel, this);
}
onTouchStart(touch) {
// 触摸开始时的逻辑处理
// 在屏幕上点击触发的事件
}
onTouchMove(touch) {
// 触摸移动时的逻辑处理
// 手指在屏幕上移动时触发的事件
}
onTouchEnd(touch) {
// 触摸结束时的逻辑处理
// 手指离开屏幕时触发的事件
}
onTouchCancel(touch) {
// 触摸取消时的逻辑处理
// 触摸意外取消时触发的事件
}
}
```
在上述代码中,使用了Cocos Creator的cc.Node的on方法来监听触摸事件。在onLoad方法中添加了cc.Node.EventType.TOUCH_START、cc.Node.EventType.TOUCH_MOVE、cc.Node.EventType.TOUCH_END和cc.Node.EventType.TOUCH_CANCEL这四个事件的监听器。然后,我们可以根据需要在对应的触摸事件处理函数(onTouchStart、onTouchMove、onTouchEnd和onTouchCancel)中编写触摸事件的逻辑处理。
通过这样的代码编写,就可以实现允许触屏的功能。当用户触摸屏幕时,相应的触摸事件处理函数将会被调用,从而实现想要的效果。
阅读全文
相关推荐
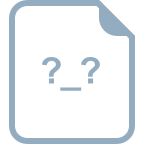
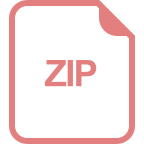
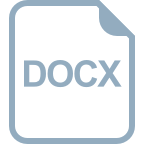
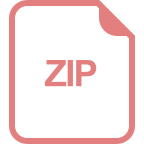
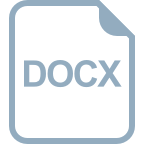
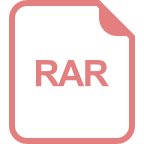
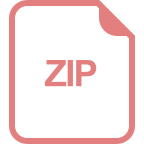
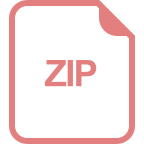
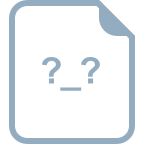
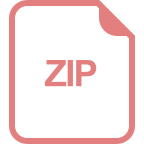
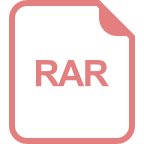
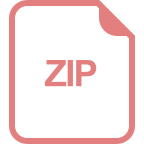
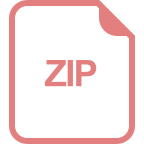
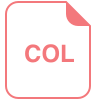
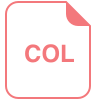

