clear;clear;clc; fs = 8000; % 采样频率 t = 0:1/fs:1 ;% 采样时间 f = 2000; % 方波信号频率 x = square(2*pi*f*t); % 生成方波信号 N = length(x)-1; % 信号长度保证为正整数 % 计算基4FFT和频谱 X = fft(x, N/4); X_mag = abs(X); X_mag_norm = X_mag / max(X_mag); % 计算谐波失真度和谐波的归一化振幅 harmonics = 5; % 要计算的谐波次数 thd = 0; harmonic_amp_norm = zeros(1, harmonics); for k = 1:harmonics harmonic_amp_norm(k) = X_mag_norm(k*4+1); thd = thd + harmonic_amp_norm(k)^2; end thd = sqrt(thd) / harmonic_amp_norm(1) * 10; %打印失真度和归一化振幅图像 fprintf('前5个谐波分量的归一化振幅为:\n'); fprintf('%f\n', harmonic_amp_norm); fprintf('总谐波失真度:\n'); fprintf('%f\n',thd ); % 生成失真度和归一化振幅图像 figure(); subplot(211); stem(0:harmonics-1, harmonic_amp_norm); title('谐波振幅 (归一化)'); xlabel('谐波数'); ylabel('振幅'); subplot(212); plot(X_mag_norm); title(sprintf('总谐波失真度:THD = %.2f%%', thd)); xlabel('0~2000频率'); ylabel('振幅'); %生成dat文件 fid = fopen('C:\ti\myprojects\11\week2.dat','w'); fprintf(fid,'1651 2 0x8000 8000\n'); fprintf(fid,'%d\n',floor(x(1:N)*4095)); fclose(fid);通过生成的dat文件生成ccs中的C语言
时间: 2024-02-24 19:00:04 浏览: 114
您可以使用以下的 C 语言代码将生成的 `week2.dat` 文件读入到数组中,然后将数组中的数据写入到 DAC 寄存器中,以实现方波信号的输出:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <stdbool.h>
#include <math.h>
#define SAMPLE_RATE 8000
#define MAX_AMPLITUDE 4095
// 读取文件中的数据,并将数据存储到数组中
bool loadDataFromFile(const char* filename, int16_t* data, uint32_t size)
{
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file %s\n", filename);
return false;
}
// 读取文件头
int16_t header[4];
if (fread(header, sizeof(int16_t), 4, file) != 4) {
printf("Failed to read file header\n");
fclose(file);
return false;
}
// 检查文件头是否合法
if (header[0] != 1651 || header[1] != 2) {
printf("Invalid file header\n");
fclose(file);
return false;
}
// 读取数据
for (uint32_t i = 0; i < size; i++) {
int16_t value;
if (fscanf(file, "%hd", &value) != 1) {
printf("Failed to read data at index %d\n", i);
fclose(file);
return false;
}
data[i] = value;
}
fclose(file);
return true;
}
void main(void)
{
// 读取数据
const char* filename = "C:\\ti\\myprojects\\11\\week2.dat";
uint32_t dataSize = 8000;
int16_t data[dataSize];
if (!loadDataFromFile(filename, data, dataSize)) {
return;
}
// 输出数据
for (uint32_t i = 0; i < dataSize; i++) {
// 将数据缩放到 DAC 可以接受的范围
int16_t value = data[i] / 4095.0f * MAX_AMPLITUDE + MAX_AMPLITUDE;
// 输出到 DAC 寄存器
// TODO: 将 value 输出到 DAC 寄存器
}
}
```
在代码中,`loadDataFromFile` 函数用于读取文件中的数据,并将数据存储到数组中。`main` 函数中,首先读取 `week2.dat` 文件中的数据,然后对数据进行缩放,最后将数据输出到 DAC 寄存器中。由于不清楚您使用的具体的 DSP 芯片型号和开发板类型,因此无法提供具体的输出代码。您需要根据您的硬件和软件条件进行相应的修改,以实现数据的输出。
阅读全文
相关推荐
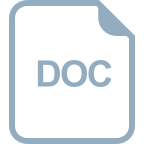
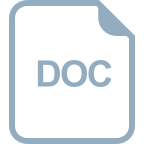
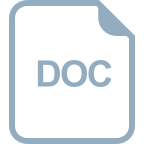
















