使用Python+Kivy编写一段Android手机的蓝牙收发程序
时间: 2024-03-01 18:49:43 浏览: 481
以下是一个简单的使用Python+Kivy编写的Android手机蓝牙收发程序示例:
```python
# 导入必要的模块
import bluetooth
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.textinput import TextInput
from kivy.uix.boxlayout import BoxLayout
class BluetoothApp(App):
def __init__(self):
super().__init__()
self.server_sock = None
self.client_sock = None
self.client_info = None
def start_server(self):
# 启动蓝牙服务端
self.server_sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
self.server_sock.bind(("", bluetooth.PORT_ANY))
self.server_sock.listen(1)
port = self.server_sock.getsockname()[1]
uuid = "00001101-0000-1000-8000-00805F9B34FB"
bluetooth.advertise_service(self.server_sock, "BluetoothServer", service_id=uuid, service_classes=[uuid, bluetooth.SERIAL_PORT_CLASS], profiles=[bluetooth.SERIAL_PORT_PROFILE])
print("等待客户端连接...")
self.client_sock, self.client_info = self.server_sock.accept()
print("客户端已连接:", self.client_info)
def stop_server(self):
# 停止蓝牙服务端
if self.client_sock:
self.client_sock.close()
self.client_sock = None
if self.server_sock:
self.server_sock.close()
self.server_sock = None
print("服务端已关闭")
def send_data(self, data):
# 发送数据
if self.client_sock:
self.client_sock.send(data.encode("utf-8"))
def receive_data(self):
# 接收数据
if self.client_sock:
data = self.client_sock.recv(1024)
return data.decode("utf-8")
return ""
def build(self):
# 构建界面
layout = BoxLayout(orientation="vertical")
# 输入框
self.text_input = TextInput()
layout.add_widget(self.text_input)
# 发送按钮
self.send_button = Button(text="发送")
self.send_button.bind(on_press=self.on_send_button_pressed)
layout.add_widget(self.send_button)
# 接收框
self.text_output = TextInput(readonly=True)
layout.add_widget(self.text_output)
# 启动按钮
self.start_button = Button(text="启动服务端")
self.start_button.bind(on_press=self.on_start_button_pressed)
layout.add_widget(self.start_button)
# 停止按钮
self.stop_button = Button(text="停止服务端")
self.stop_button.bind(on_press=self.on_stop_button_pressed)
layout.add_widget(self.stop_button)
return layout
def on_start_button_pressed(self, instance):
# 启动服务端
self.start_server()
def on_stop_button_pressed(self, instance):
# 停止服务端
self.stop_server()
def on_send_button_pressed(self, instance):
# 发送数据
data = self.text_input.text
self.send_data(data)
def on_update(self, dt):
# 更新接收框
data = self.receive_data()
if data:
self.text_output.text += data
BluetoothApp().run()
```
这段程序实现了一个简单的蓝牙收发应用,包括启动、停止服务端、发送数据和接收数据等功能。在界面中,可以输入要发送的数据并通过“发送”按钮发送出去,同时,接收到的数据也会显示在接收框中。同时,该应用也提供了服务端的启动和停止按钮,可以方便地控制服务端的启停。
阅读全文
相关推荐
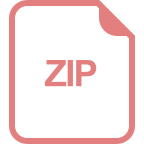
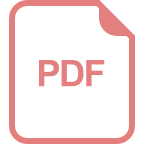
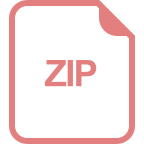















