卷积神经网络中怎么添加注意力机制python代码
时间: 2023-10-23 19:13:05 浏览: 237
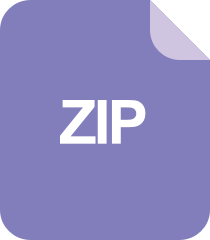
本科毕设-基于注意力机制和图卷积神经网络的多任务谣言检测python源码+数据集.zip

在卷积神经网络中添加注意力机制,可以通过以下代码实现:
```python
import tensorflow as tf
class AttentionLayer(tf.keras.layers.Layer):
def __init__(self, **kwargs):
super(AttentionLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.W = self.add_weight(name="att_weight", shape=(input_shape[-1], 1), initializer="normal")
self.b = self.add_weight(name="att_bias", shape=(input_shape[1], 1), initializer="zeros")
super(AttentionLayer, self).build(input_shape)
def call(self, x):
e = tf.keras.backend.dot(x, self.W) + self.b
a = tf.keras.backend.softmax(e, axis=1)
output = x * a
return tf.keras.backend.sum(output, axis=1)
```
以上代码实现了一个自定义的 AttentionLayer 类,通过该类可以将注意力机制加入到卷积神经网络中。在该类中,`build` 方法用于构建权重,`call` 方法用于计算注意力向量并输出加权后的特征向量。
使用该 AttentionLayer 类,可以在卷积神经网络中添加注意力机制,具体代码如下:
```python
import tensorflow as tf
input_layer = tf.keras.layers.Input(shape=(32, 32, 3))
conv_layer = tf.keras.layers.Conv2D(filters=64, kernel_size=(3, 3), activation="relu")(input_layer)
pooling_layer = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(conv_layer)
flatten_layer = tf.keras.layers.Flatten()(pooling_layer)
attention_layer = AttentionLayer()(flatten_layer)
output_layer = tf.keras.layers.Dense(units=10, activation="softmax")(attention_layer)
model = tf.keras.models.Model(inputs=input_layer, outputs=output_layer)
```
以上代码实现了一个简单的卷积神经网络,其中包含一个卷积层、一个池化层、一个展平层、一个自定义的 AttentionLayer 层和一个全连接层。在 AttentionLayer 层后面使用了一个全连接层,用于最终的分类任务。
阅读全文
相关推荐
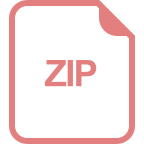
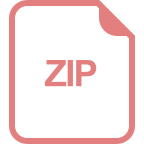















