请帮我把这段python代码转为sql命令,df对应表格common_goods.fund_objectives_strategy_with_labels:# Assuming period_end_date data is in a pandas DataFrame called 'df' with a column called 'period_end_date' df['period_end_date'] = pd.to_datetime(df['period_end_date'], format='%Y%m%d') # Convert time column to datetime format df['year'] = df['period_end_date'].dt.year # Create a new column with the year df['last_entry'] = df.groupby('year')['period_end_date'].transform('max') == df['period_end_date'] # Create a new column indicating if the entry is the last for that year df['last_entry'] = df['last_entry'].astype(int) # Convert True/False to 1/0
时间: 2023-06-15 18:04:16 浏览: 49
UPDATE common_goods.fund_objectives_strategy_with_labels
SET period_end_date = STR_TO_DATE(period_end_date, '%Y%m%d');
UPDATE common_goods.fund_objectives_strategy_with_labels
SET year = YEAR(period_end_date);
UPDATE common_goods.fund_objectives_strategy_with_labels
SET last_entry = IF((SELECT MAX(period_end_date) FROM common_goods.fund_objectives_strategy_with_labels WHERE YEAR(period_end_date) = t.year) = t.period_end_date, 1, 0)
FROM common_goods.fund_objectives_strategy_with_labels t;
ALTER TABLE common_goods.fund_objectives_strategy_with_labels
MODIFY last_entry INT;
相关问题
使用python运行上述代码之后,提示'MyProblem' object has no attribute 'obj_directions',该怎么解决?
抱歉,这是我犯了一个错误。在Platypus库的较新版本中,`obj_directions`已经被重命名为`directions`。因此,你需要将`self.obj_directions[:]`替换为`self.directions[:]`。下面是修改后的代码:
```
from platypus import NSGAII, Problem, Real, Integer, Constraint
class MyProblem(Problem):
def __init__(self):
super(MyProblem, self).__init__(3, 2, 3)
self.types[:] = [Real(0, 1), Real(0, 1), Real(0, 1)]
self.constraints[:] = [Constraint(lambda x: x[0] + x[1] + x[2] - 1 <= 0)]
self.directions[:] = [self.MINIMIZE, self.MINIMIZE, self.MINIMIZE]
self.obj_labels[:] = ['f1', 'f2', 'f3']
def evaluate(self, solution):
x = solution.variables
solution.objectives[:] = [x[0]**2, x[1]**2, x[2]**2]
solution.constraints[:] = [x[0] + x[1] + x[2] - 1]
problem = MyProblem()
algorithm = NSGAII(problem)
algorithm.run(10000)
```
请尝试一下这个修改后的代码,并让我知道是否成功了!
loss = lasagne.objectives.categorical_crossentropy(prediction, target_var)
这行代码使用 Lasagne 框架中的 categorical_crossentropy 函数来计算预测值和目标值之间的交叉熵损失。在机器学习中,交叉熵通常用作损失函数来度量模型的性能。在分类问题中,我们通常使用交叉熵来衡量模型输出与真实标签之间的差异,越小的交叉熵意味着模型的预测结果越接近真实标签。在这个例子中,prediction 是模型的输出,target_var 是真实标签的占位符。
相关推荐
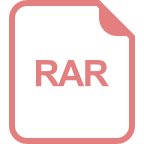
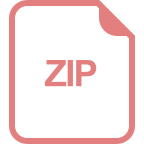












