unity2d平台跳跃
时间: 2023-11-17 20:08:33 浏览: 31
在 Unity 2D 中实现跳跃需要以下步骤:
1. 给角色添加刚体组件,并设置重力为正向下的值。
2. 给角色添加碰撞体,并设置为合适的大小和形状。
3. 在角色的脚下添加一个空物体作为检测是否着地的点。
4. 编写脚本,在 Update 函数中检测是否按下跳跃键,如果按下则给角色一个向上的力。
5. 在脚本中检测角色是否着地,如果着地则可以再次跳跃,否则不能跳跃。
以下是一个示例脚本:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float jumpForce = 10f; // 跳跃力度
public Transform groundCheck; // 检测是否着地的点
public float groundRadius = 0.2f; // 检测是否着地的半径
public LayerMask whatIsGround; // 着地的 Layer
private Rigidbody2D rb;
private bool isGrounded = false;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
isGrounded = Physics2D.OverlapCircle(groundCheck.position, groundRadius, whatIsGround);
}
void Update()
{
if (isGrounded && Input.GetKeyDown(KeyCode.Space))
{
rb.AddForce(Vector2.up * jumpForce, ForceMode2D.Impulse);
}
}
}
```
在该脚本中,我们使用了 OverlapCircle 函数来检测是否着地。在 FixedUpdate 函数中更新 isGrounded 变量的值。在 Update 函数中检测是否按下跳跃键,如果按下则给角色一个向上的力。如果 isGrounded 为 true,则可以再次跳跃。
相关推荐














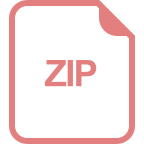