如何使用celery定时任务,批量推送邮件
时间: 2023-07-18 16:24:37 浏览: 71
使用Celery可以很方便地实现定时任务和批量推送邮件。以下是一个示例代码,演示如何使用Celery定时发送批量邮件:
1. 安装Celery和Redis
```bash
pip install celery redis
```
2. 创建Celery实例
```python
# tasks.py
from celery import Celery
app = Celery('tasks', broker='redis://localhost:6379/0')
```
3. 编写发送邮件的函数
```python
# tasks.py
import smtplib
from email.mime.text import MIMEText
@app.task
def send_email(recipient, subject, body):
# 创建邮件内容
message = MIMEText(body)
message['From'] = 'sender@example.com'
message['To'] = recipient
message['Subject'] = subject
# 连接邮件服务器
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login('username', 'password')
# 发送邮件
server.sendmail('sender@example.com', recipient, message.as_string())
# 关闭连接
server.quit()
```
4. 编写定时任务
```python
# tasks.py
from datetime import datetime, timedelta
@app.task
def send_batch_emails():
# 构造邮件列表
recipients = ['user1@example.com', 'user2@example.com', 'user3@example.com']
subject = 'Test Email'
body = 'This is a test email.'
# 发送邮件
for recipient in recipients:
send_email.apply_async(args=[recipient, subject, body])
@app.task
def send_periodic_emails():
# 每天10点发送一次邮件
schedule_time = datetime.now().replace(hour=10, minute=0, second=0)
if schedule_time < datetime.now():
schedule_time += timedelta(days=1)
send_batch_emails.apply_async(eta=schedule_time)
```
5. 启动Celery
```bash
celery -A tasks worker --loglevel=info
```
6. 定时发送邮件
```python
# 在任意位置调用 send_periodic_emails() 函数即可
from tasks import send_periodic_emails
send_periodic_emails.delay()
```
在上面的代码中,我们使用Celery创建了一个异步任务send_email(),用于发送单个邮件。然后,我们创建了一个异步任务send_batch_emails(),用于批量发送邮件。send_batch_emails()函数遍历收件人列表,为每个收件人创建一个send_email任务,并异步发送邮件。
最后,我们创建了一个定时任务send_periodic_emails(),用于每天10点发送一次邮件。我们使用Celery的apply_async()方法设置任务的执行时间,并在启动Celery后调用该函数即可。
您可以根据自己的需要修改邮件内容、收件人列表和定时任务的时间,并使用类似的方法来实现定时发送批量邮件。
相关推荐
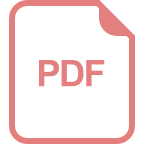
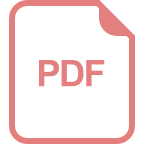














