Java 定义超类,并通过继承定义子类,在子类中重写方法
时间: 2023-12-27 13:02:08 浏览: 88
Java 中定义超类可以使用关键字 `class`,并在类名后加上 `extends` 关键字和超类的名称,例如:
```
public class SuperClass {
// 超类的属性和方法
}
public class SubClass extends SuperClass {
// 子类的属性和方法
}
```
在子类中重写超类的方法,可以使用 `@Override` 注解来标记方法,确保子类中的方法与超类中的方法签名一致,例如:
```
public class SuperClass {
public void doSomething() {
// 超类的实现
}
}
public class SubClass extends SuperClass {
@Override
public void doSomething() {
// 子类的实现
}
}
```
这样,在使用子类对象调用 `doSomething()` 方法时,会优先执行子类中重写的方法。
相关问题
Java定义超类,并通过继承定义子类,在子类中重写方法
在Java中,定义超类和子类并重写方法是面向对象编程的核心概念之一。以下是如何在Java中定义超类、通过继承定义子类以及在子类中重写方法的步骤和示例:
### 定义超类
首先,我们定义一个超类,例如`Animal`:
```java
public class Animal {
// 超类中的方法
public void makeSound() {
System.out.println("Animal makes a sound");
}
}
```
### 定义子类并重写方法
接下来,我们定义一个子类,例如`Dog`,并重写`makeSound`方法:
```java
public class Dog extends Animal {
// 子类中重写的方法
@Override
public void makeSound() {
System.out.println("Dog barks");
}
}
```
### 使用子类
最后,我们可以在主类中使用这些类:
```java
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Animal();
Animal myDog = new Dog();
myAnimal.makeSound(); // 输出: Animal makes a sound
myDog.makeSound(); // 输出: Dog barks
}
}
```
### 解释
1. **超类定义**:在`Animal`类中,我们定义了一个`makeSound`方法。
2. **子类继承**:在`Dog`类中,我们通过`extends`关键字继承自`Animal`类。
3. **方法重写**:在`Dog`类中,我们重写了`makeSound`方法,使用`@Override`注解来表明这是一个重写的方法。
4. **对象创建**:在`Main`类的`main`方法中,我们创建了`Animal`和`Dog`的对象,并调用`makeSound`方法。可以看到,`Dog`对象调用的是重写后的方法。
Java 定义超类,并通过继承定义子类,在子类中重写方法代码
以下是一个例子:
```java
// 定义超类 Animal
public class Animal {
public void makeSound() {
System.out.println("This is the sound of an animal.");
}
}
// 定义子类 Cat,继承 Animal
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("The cat meows.");
}
}
```
在这个例子中,超类 Animal 定义了一个 makeSound 方法,该方法输出一个字符串。子类 Cat 继承了超类 Animal 并重写了 makeSound 方法,将输出改为“猫叫”。
注意,在子类中重写超类的方法时,需要使用 `@Override` 注解来明确表示该方法是对超类方法的重写。这样有助于编译器检查方法签名是否正确,并且可以使代码更易于理解。
阅读全文
相关推荐
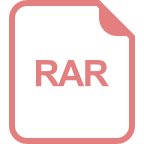












