如何在carla中设置4个NPC车辆,并将这4个NPC车辆的实时速度与玩家车辆之间的距离放在一个新的csv表里
时间: 2024-03-17 14:40:00 浏览: 14
要在CARLA中设置四辆NPC汽车,需要创建四个蓝图。可以使用CARLA提供的Python API来完成这个任务。以下是一个简单的代码示例:
``` python
import carla
client = carla.Client('localhost', 2000)
client.set_timeout(2.0)
world = client.get_world()
blueprints = world.get_blueprint_library()
# 获取NPC车辆蓝图
vehicle_bp = blueprints.filter('vehicle.*')
# 设置NPC车辆初始位置
spawn_points = world.get_map().get_spawn_points()
spawn_point = spawn_points[0]
# 生成四辆NPC车辆
batch = []
for i in range(4):
transform = carla.Transform(spawn_point.location + carla.Location(x=i*4.0, y=0, z=0),
spawn_point.rotation)
batch.append(carla.command.SpawnActor(vehicle_bp.randomize_color(), transform))
# 发送生成NPC车辆的请求
responses = client.apply_batch_sync(batch)
# 存储NPC车辆的actor_id
actor_ids = []
for response in responses:
if response.error:
print(response.error)
else:
actor_ids.append(response.actor_id)
# 将NPC车辆的actor_id存储到CSV表中
import csv
with open('actor_ids.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['actor_id'])
for actor_id in actor_ids:
writer.writerow([actor_id])
```
要在CARLA中获取NPC车辆的实时速度和与玩家车辆之间的距离,可以使用CARLA提供的Python API。以下是一个简单的代码示例:
``` python
import carla
import csv
client = carla.Client('localhost', 2000)
client.set_timeout(2.0)
world = client.get_world()
# 获取玩家车辆和NPC车辆的actor_id
player_actor_id = world.player.id
npc_actor_ids = []
with open('actor_ids.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
next(reader) # 跳过头部
for row in reader:
npc_actor_ids.append(int(row[0]))
# 创建实时速度和距离的CSV表
with open('speed_distance.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['frame', 'player_speed', 'npc_speeds', 'distances'])
# 循环采集数据
frame = 0
while True:
# 获取玩家车辆的速度
player_velocity = world.get_actor(player_actor_id).get_velocity()
player_speed = carla.Vector3D(player_velocity.x, player_velocity.y, player_velocity.z).length()
# 获取NPC车辆的速度和距离
npc_speeds = []
distances = []
for npc_actor_id in npc_actor_ids:
npc_velocity = world.get_actor(npc_actor_id).get_velocity()
npc_speed = carla.Vector3D(npc_velocity.x, npc_velocity.y, npc_velocity.z).length()
npc_speeds.append(npc_speed)
player_location = world.get_actor(player_actor_id).get_location()
npc_location = world.get_actor(npc_actor_id).get_location()
distance = carla.Vector3D(player_location.x - npc_location.x,
player_location.y - npc_location.y,
player_location.z - npc_location.z).length()
distances.append(distance)
# 将数据存储到CSV表中
with open('speed_distance.csv', 'a', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow([frame, player_speed] + npc_speeds + distances)
frame += 1
```
这段代码将会循环采集玩家车辆和NPC车辆的速度以及它们之间的距离,并将数据存储到一个CSV表中。你可以使用Excel或其它工具来分析这些数据。
相关推荐
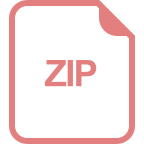
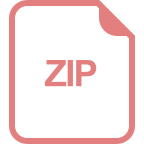














