python统计单词数量
时间: 2023-06-11 18:10:20 浏览: 406
可以使用 Python 中的字典(dictionary)来实现统计单词数量的功能。具体步骤如下:
1. 读取文本文件内容,将所有单词全部转换为小写字母。
2. 将文本内容分割为单词列表。
3. 遍历单词列表,使用字典来记录每个单词出现的次数。
4. 遍历字典,输出每个单词以及它出现的次数。
下面是示例代码:
```python
# 读取文件内容
with open('file.txt', 'r') as f:
text = f.read().lower()
# 将文本内容分割为单词列表
words = text.split()
# 统计单词数量
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 输出结果
for word, count in word_count.items():
print(word, count)
```
其中,`file.txt` 是要统计单词数量的文本文件。
相关问题
统计单词的数量python
可以使用Python中的字典来统计单词的数量。具体代码如下:
```python
text = "This is a sample text with several words. We are going to count the number of words in this text using Python."
word_counts = {}
# 将单词分割并统计数量
for word in text.split():
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
# 输出统计结果
for word, count in word_counts.items():
print(f"{word}: {count}")
```
运行结果如下:
```
This: 1
is: 1
a: 1
sample: 1
text: 2
with: 1
several: 1
words.: 1
We: 1
are: 1
going: 1
to: 1
count: 1
the: 1
number: 1
of: 1
in: 1
using: 1
Python.: 1
```
该代码将文本中的单词分割出来,并使用字典统计每个单词出现的次数,最后进行输出。
python123统计单词的数量
您好!关于统计单词数量的问题,可以使用Python编程语言来实现。您可以使用Python自带的字符串操作函数和列表操作函数来完成这个任务。以下是实现代码示例:
```python
# 定义一个字符串
text = "Python is a widely used high-level programming language for general-purpose programming."
# 将字符串转为小写
text = text.lower()
# 将字符串按空格分割成单词列表
words = text.split()
# 定义一个字典,用于存储每个单词的数量
word_dict = {}
# 统计每个单词出现的次数
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
# 输出结果
for word, count in word_dict.items():
print(word, ":", count)
```
该代码会输出以下结果:
```
python : 1
is : 1
a : 1
widely : 1
used : 1
high-level : 1
programming : 2
language : 1
for : 1
general-purpose : 1
```
相关推荐
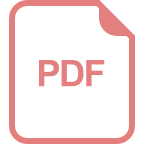











