android 取消蓝牙配对代码
时间: 2023-07-30 13:10:54 浏览: 45
在 Android 中,可以通过 BluetoothAdapter 类的 unpairDevice() 方法来取消蓝牙配对。具体代码如下:
```
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress); // deviceAddress 为需要取消配对的蓝牙设备地址
// 取消配对
Method method = device.getClass().getMethod("removeBond", (Class[]) null);
method.invoke(device, (Object[]) null);
```
需要注意的是,该方法需要在 Android 2.3 及以上版本中才能使用。同时,需要在 AndroidManifest.xml 文件中添加蓝牙相关的权限:
```
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
```
相关问题
android 取消蓝牙配对
在 Android 上取消蓝牙配对,可以按照以下步骤进行操作:
1. 打开 Android 设备的蓝牙设置,找到已经配对的蓝牙设备。
2. 点击需要取消配对的蓝牙设备,进入设备详情页面。
3. 在设备详情页面中,找到取消配对的选项,一般为“取消配对”或“忘记设备”。
4. 点击取消配对按钮,等待一段时间后,设备的配对信息就会被删除。
注意:取消配对后,设备之间的连接也会断开,如果需要重新连接,需要重新进行配对操作。
android蓝牙取消配对弹窗
在Android系统中,要取消与蓝牙设备的配对,可以通过以下步骤弹出取消配对的弹窗:
1. 打开蓝牙设置:在手机的设置菜单中找到“蓝牙”选项,并点击进入。
2. 查找已配对设备列表:在蓝牙设置界面中,会显示已经配对的蓝牙设备列表,可以滚动查看。
3. 选择要取消配对的设备:在已配对设备列表中找到想要取消配对的设备,并点击设备名称。
4. 弹出取消配对弹窗:点击设备名称后,会弹出一个新的窗口,该窗口会显示设备的详细信息和连接状态,同时也会显示一个“取消配对”或“解除配对”的按钮。
5. 确认取消配对:点击“取消配对”或“解除配对”按钮后,系统会提示是否确认取消配对。如果确认取消配对,则取消成功,设备将从已配对列表中移除,并断开与该设备的连接。
需要注意的是,不同Android版本和设备厂商的界面可能有所差异,但大致的步骤和操作方式应该是类似的。此外,有些设备在取消配对时可能会要求输入配对码或进行验证操作,请根据具体提示进行操作。
总结:要在Android系统中取消蓝牙设备的配对,首先进入蓝牙设置界面,然后找到并选择要取消配对的设备,在设备详细信息界面点击“取消配对”或“解除配对”按钮,最后确认取消配对。
相关推荐
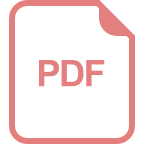












