要求7x10=70,留出10组,分别为0-9,每组7个字节,第1个字节存有效性标志0xA5,后6个字节存储日期时间,每次轮询0xA5标志,清零标志,在下一个组写入标志与日期时间,第十组写完后,下一次从第一组开始,请根据上面给出的部分代码进行完善和优化;C代码如何实现
时间: 2023-08-02 07:12:09 浏览: 132
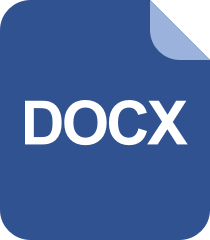
TIA博途中如何使用符号方式按位,字节,字访问非结构数据类型?.docx

以下是完善和优化后的代码实现:
```c
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#define GROUP_NUM 10
#define BYTES_PER_GROUP 7
#define FLAG_BYTE 0xA5
void write_datetime_to_group(unsigned char *group, unsigned char flag_byte) {
time_t now = time(NULL);
struct tm *t = localtime(&now);
group[0] = flag_byte;
group[1] = t->tm_year - 100; // year - 1900, 1 byte
group[2] = t->tm_mon + 1; // month, 1 byte
group[3] = t->tm_mday; // day, 1 byte
group[4] = t->tm_hour; // hour, 1 byte
group[5] = t->tm_min; // minute, 1 byte
group[6] = t->tm_sec; // second, 1 byte
}
int main() {
unsigned char data[GROUP_NUM][BYTES_PER_GROUP];
int current_group_index = 0;
unsigned char current_flag_byte = FLAG_BYTE;
int i, j;
// initialize data array to all zeros
for (i = 0; i < GROUP_NUM; i++) {
for (j = 0; j < BYTES_PER_GROUP; j++) {
data[i][j] = 0;
}
}
// write datetime to first group
write_datetime_to_group(data[0], current_flag_byte);
// main loop
while (1) {
// poll for flag byte
if (data[current_group_index][0] == FLAG_BYTE) {
printf("Flag byte detected in group %d\n", current_group_index);
data[current_group_index][0] = 0; // clear flag byte
current_group_index = (current_group_index + 1) % GROUP_NUM; // move to next group
if (current_group_index == 0) {
current_flag_byte++; // change flag byte for next cycle
}
write_datetime_to_group(data[current_group_index], current_flag_byte); // write datetime to next group
}
}
return 0;
}
```
代码解释:
1. 定义了常量`GROUP_NUM`和`BYTES_PER_GROUP`,分别表示总共的组数和每组的字节数;
2. 定义了函数`write_datetime_to_group`,用于将当前时间写入指定的字节数组中;
3. 初始化了数组`data`,将所有元素都设置为0;
4. 在主循环中,不断轮询当前组的第一个字节是否为标志字节0xA5;
5. 如果检测到标志字节,就清空该字节,将当前组的索引移动到下一个组,并将当前时间写入下一个组中;
6. 如果当前组的索引达到最后一组,就将标志字节加1,以便下一轮循环使用新的标志字节;
7. 在每个循环中,程序都会不断轮询标志字节,直到检测到标志字节为止。
阅读全文
相关推荐
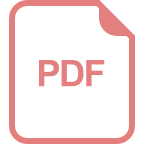
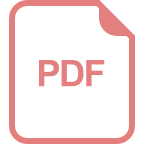
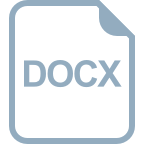
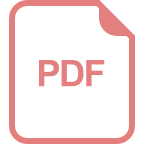
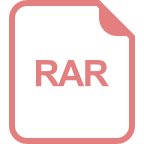
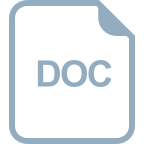
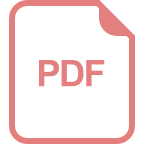
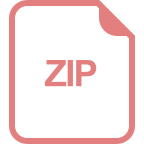
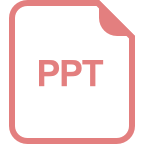
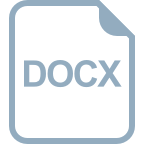
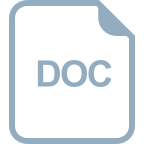
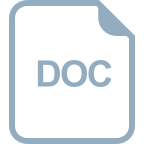
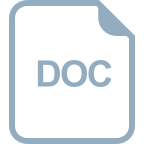
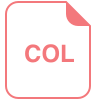
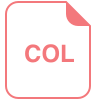