c语言查找字符串括号中间的字符串
时间: 2023-06-21 16:08:04 浏览: 98
可以利用栈来实现查找字符串括号中间的字符串。具体步骤如下:
1. 定义一个栈,用于存储左括号的下标。
2. 遍历字符串,遇到左括号时,将其下标入栈。
3. 遇到右括号时,弹出栈顶元素,该元素对应的下标即为左括号的下标。
4. 截取左括号和右括号之间的子串,即为括号中间的字符串。
以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
#define STACK_SIZE 100
int stack[STACK_SIZE];
int top = -1;
void push(int x)
{
if (top == STACK_SIZE - 1) {
printf("Stack overflow!\n");
return;
}
stack[++top] = x;
}
int pop()
{
if (top == -1) {
printf("Stack underflow!\n");
return -1;
}
return stack[top--];
}
int main()
{
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == '(') {
push(i);
} else if (str[i] == ')') {
int left = pop();
if (left != -1) {
printf("Substring between parentheses: %.*s\n", i - left - 1, str + left + 1);
}
}
}
return 0;
}
```
输入示例:
```
Enter a string: hello(world)how(are(you))
```
输出示例:
```
Substring between parentheses: world
Substring between parentheses: are
Substring between parentheses: you
```
阅读全文
相关推荐
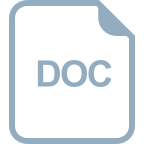
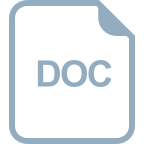
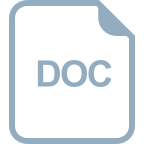
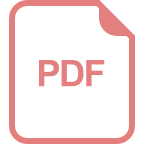
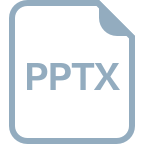
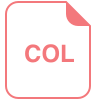
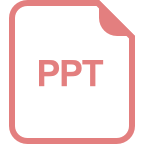
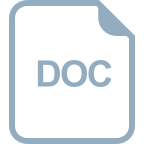
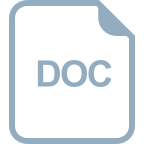
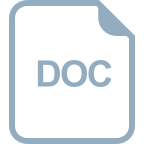
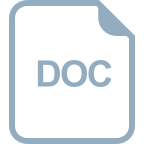
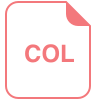
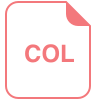





