黄金矿工 C++ 多文件 easyx
时间: 2023-08-05 09:07:31 浏览: 99
好的,黄金矿工的C++多文件版本需要使用EasyX图形库,以下是一个简单的示例代码:
main.cpp:
```cpp
#include "graphics.h"
#include "game.h"
int main()
{
initgraph(WIDTH, HEIGHT); // 初始化图形界面
Game game; // 创建游戏对象
game.run(); // 运行游戏
closegraph(); // 关闭图形界面
return 0;
}
```
game.h:
```cpp
#ifndef GAME_H
#define GAME_H
#include "object.h"
class Game {
public:
Game();
~Game();
void run(); // 游戏主循环
private:
Object* player; // 玩家对象
Object* gold; // 黄金对象
int score; // 得分
};
#endif
```
game.cpp:
```cpp
#include "game.h"
#include "object.h"
Game::Game()
{
player = new Object(PLAYER_X, PLAYER_Y, PLAYER_SIZE, PLAYER_COLOR); // 创建玩家对象
gold = new Object(GOLD_X, GOLD_Y, GOLD_SIZE, GOLD_COLOR); // 创建黄金对象
score = 0; // 初始化得分
}
Game::~Game()
{
delete player; // 释放玩家对象内存
delete gold; // 释放黄金对象内存
}
void Game::run()
{
while (true) {
cleardevice(); // 清空画面
player->draw(); // 绘制玩家
gold->draw(); // 绘制黄金
if (player->intersect(*gold)) { // 碰撞检测
score += 10; // 加分
gold->move(); // 移动黄金
}
player->move(); // 移动玩家
settextcolor(WHITE); // 设置文字颜色
settextstyle(20, 0, _T("Consolas")); // 设置文字样式
TCHAR str[64];
_stprintf_s(str, _T("得分:%d"), score); // 格式化字符串
outtextxy(10, 10, str); // 输出文字
Sleep(FRAME_RATE); // 控制帧率
}
}
```
object.h:
```cpp
#ifndef OBJECT_H
#define OBJECT_H
#include "graphics.h"
class Object {
public:
Object(int x, int y, int size, COLORREF color);
~Object();
void draw(); // 绘制对象
void move(); // 移动对象
bool intersect(Object& other); // 碰撞检测
private:
int x; // x坐标
int y; // y坐标
int size; // 大小
COLORREF color; // 颜色
int vx; // x方向速度
int vy; // y方向速度
};
#endif
```
object.cpp:
```cpp
#include "object.h"
Object::Object(int x, int y, int size, COLORREF color)
{
this->x = x;
this->y = y;
this->size = size;
this->color = color;
vx = 0;
vy = 0;
}
Object::~Object()
{
}
void Object::draw()
{
setfillcolor(color);
fillcircle(x, y, size);
}
void Object::move()
{
x += vx;
y += vy;
if (x < 0 || x > WIDTH) { // 边界检测
vx = -vx; // 反弹
}
if (y < 0 || y > HEIGHT) { // 边界检测
vy = -vy; // 反弹
}
}
bool Object::intersect(Object& other)
{
int dx = x - other.x;
int dy = y - other.y;
int d = size + other.size;
return dx * dx + dy * dy <= d * d;
}
```
在上述代码中,main.cpp是程序入口,通过调用Game对象的run方法来运行游戏。Game类中包含玩家对象、黄金对象和得分三个成员变量,run方法是游戏的主循环,每帧处理玩家移动、黄金移动和碰撞检测等逻辑,并绘制游戏界面。Object类是游戏中的所有可移动对象的基类,包含了绘制、移动和碰撞检测等方法。
相关推荐
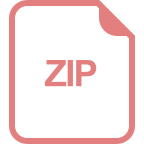






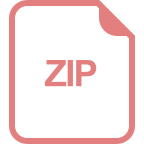
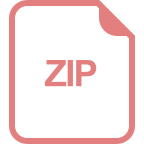
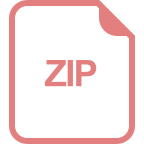
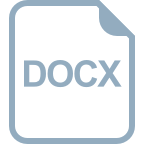