定义一个结构体变量(包括年、月、日)。输入一个日期,输出该日的下一天的日期
时间: 2023-06-17 15:08:14 浏览: 115
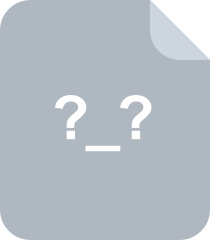
1.定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年的问题。
以下是一个C++的实现:
```cpp
#include <iostream>
using namespace std;
struct Date {
int year;
int month;
int day;
};
// 判断是否是闰年
bool isLeapYear(int year) {
return year % 400 == 0 || (year % 4 == 0 && year % 100 != 0);
}
// 获取某个月份的天数
int getDaysOfMonth(int year, int month) {
switch (month) {
case 2:
return isLeapYear(year) ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
// 获取某个日期的下一天日期
Date getNextDay(Date date) {
int days = getDaysOfMonth(date.year, date.month);
if (date.day < days) {
date.day++;
} else {
date.day = 1;
if (date.month < 12) {
date.month++;
} else {
date.month = 1;
date.year++;
}
}
return date;
}
int main() {
Date date;
cout << "请输入日期(格式:年 月 日):" << endl;
cin >> date.year >> date.month >> date.day;
Date nextDay = getNextDay(date);
cout << "下一天的日期是:" << nextDay.year << "年" << nextDay.month << "月" << nextDay.day << "日" << endl;
return 0;
}
```
运行程序,输入日期后,即可输出该日期的下一天日期。
阅读全文
相关推荐
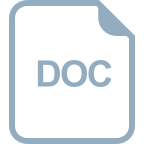














