请用python设计一个俄罗斯方块游戏,必须使用Tkinter 用户使用左、右和下箭头来控制盒子的左、右和向下移动;使用上箭头来改变正方形的方向。在游戏结束时将会显示一个弹出式对话框
时间: 2024-01-22 20:20:25 浏览: 146
好的,我可以为你提供一个简单的俄罗斯方块游戏的代码示例,使用了Tkinter实现GUI界面和键盘事件处理:
```python
import random
import tkinter as tk
from tkinter import messagebox
# 游戏区域大小(单位:像素)
CELL_SIZE = 30
BOARD_WIDTH = 10
BOARD_HEIGHT = 20
# 方块的类型和形状定义
SHAPES = [
((0,0), (1,0), (0,1), (1,1)), # 正方形
((0,0), (1,0), (2,0), (3,0)), # 长条形
((0,0), (0,1), (1,1), (2,1)), # L形
((2,0), (0,1), (1,1), (2,1)), # 反L形
((0,0), (0,1), (1,0), (2,0)), # Z形
((0,0), (1,0), (1,1), (2,1)), # 反Z形
((1,0), (0,1), (1,1), (2,1)), # T形
]
# 颜色定义
COLORS = [
'cyan', 'blue', 'orange', 'yellow', 'green', 'purple', 'red'
]
class TetrisApp:
def __init__(self, master):
self.master = master
self.master.title('俄罗斯方块')
self.master.bind('<Key>', self.key_pressed)
self.score = 0
self.board = [[0] * BOARD_WIDTH for _ in range(BOARD_HEIGHT)]
self.init_gui()
self.new_game()
def init_gui(self):
# 初始化游戏区域
self.canvas = tk.Canvas(self.master, width=CELL_SIZE * BOARD_WIDTH,
height=CELL_SIZE * BOARD_HEIGHT, bg='white')
self.canvas.pack()
# 初始化得分区域
self.score_label = tk.Label(self.master, text='得分:0', font=('Arial', 16))
self.score_label.pack()
def new_game(self):
self.score = 0
self.board = [[0] * BOARD_WIDTH for _ in range(BOARD_HEIGHT)]
self.current_shape = self.get_new_shape()
self.draw_board()
self.draw_shape()
self.master.after(500, self.game_loop)
def game_loop(self):
if not self.move_shape(0, 1):
# 方块无法下移,将其固定在游戏区域中
self.fix_shape()
# 消除满行
full_rows = self.get_full_rows()
if len(full_rows) > 0:
self.remove_rows(full_rows)
# 判断游戏是否结束
if self.is_game_over():
self.show_game_over_dialog()
return
# 生成新方块
self.current_shape = self.get_new_shape()
self.draw_shape()
self.master.after(500, self.game_loop)
def key_pressed(self, event):
if event.keysym == 'Left':
self.move_shape(-1, 0)
elif event.keysym == 'Right':
self.move_shape(1, 0)
elif event.keysym == 'Down':
self.move_shape(0, 1)
elif event.keysym == 'Up':
self.rotate_shape()
def draw_board(self):
self.canvas.delete('cell')
for i in range(BOARD_HEIGHT):
for j in range(BOARD_WIDTH):
if self.board[i][j] > 0:
x1, y1 = j * CELL_SIZE, i * CELL_SIZE
x2, y2 = x1 + CELL_SIZE, y1 + CELL_SIZE
self.canvas.create_rectangle(x1, y1, x2, y2, fill=COLORS[self.board[i][j] - 1], tags='cell')
def draw_shape(self):
self.canvas.delete('shape')
for i, j in self.current_shape:
x1, y1 = (j + self.current_col) * CELL_SIZE, (i + self.current_row) * CELL_SIZE
x2, y2 = x1 + CELL_SIZE, y1 + CELL_SIZE
self.canvas.create_rectangle(x1, y1, x2, y2, fill=COLORS[self.current_shape_type], tags='shape')
def move_shape(self, dx, dy):
'''移动方块'''
if self.check_collision(self.current_shape, self.current_row + dy, self.current_col + dx):
self.current_row += dy
self.current_col += dx
return True
else:
return False
def rotate_shape(self):
'''旋转方块'''
old_shape = self.current_shape
new_shape = [(j, -i) for i, j in old_shape]
if self.check_collision(new_shape, self.current_row, self.current_col):
self.current_shape = new_shape
return True
else:
return False
def fix_shape(self):
'''固定方块'''
for i, j in self.current_shape:
row, col = self.current_row + i, self.current_col + j
self.board[row][col] = self.current_shape_type + 1
def get_full_rows(self):
'''获取满行的行号'''
full_rows = []
for i in range(BOARD_HEIGHT):
if all(self.board[i][j] > 0 for j in range(BOARD_WIDTH)):
full_rows.append(i)
return full_rows
def remove_rows(self, rows):
'''消除满行'''
self.score += len(rows) * 100
self.score_label.config(text='得分:%d' % self.score)
for row in rows:
self.board.pop(row)
self.board.insert(0, [0] * BOARD_WIDTH)
def is_game_over(self):
'''判断游戏是否结束'''
return any(self.board[0][j] > 0 for j in range(BOARD_WIDTH))
def show_game_over_dialog(self):
'''显示游戏结束对话框'''
messagebox.showinfo('游戏结束', '得分:%d' % self.score)
self.new_game()
def check_collision(self, shape, row, col):
'''检查方块是否与游戏区域或已有方块碰撞'''
for i, j in shape:
r, c = row + i, col + j
if not (0 <= r < BOARD_HEIGHT and 0 <= c < BOARD_WIDTH and self.board[r][c] == 0):
return False
return True
def get_new_shape(self):
'''生成新方块'''
shape_type = random.randint(0, len(SHAPES) - 1)
return SHAPES[shape_type], shape_type
if __name__ == '__main__':
root = tk.Tk()
app = TetrisApp(root)
root.mainloop()
```
运行代码后,会显示一个简单的俄罗斯方块游戏界面,你可以使用左、右和下箭头来控制盒子的左、右和向下移动,使用上箭头来改变正方形的方向。在游戏结束时将会显示一个弹出式对话框,告诉你得分情况。
阅读全文
相关推荐
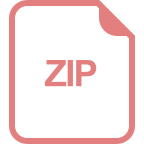
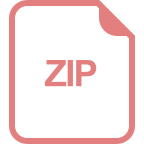



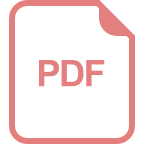

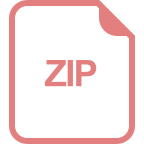
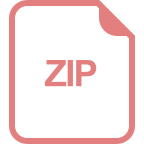
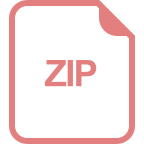
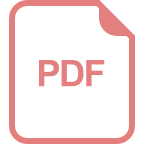
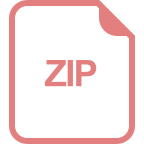
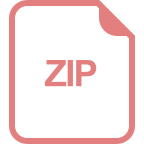