请使用python tkinter开发一个俄罗斯方块游戏,包括:为了实现基本的俄罗斯方块功能,用户使用左、右和下箭头来控制盒子的左、右和向下移动;使用上箭头来改变正方形的方向。在游戏结束时将会显示一个弹出式对话框
时间: 2024-02-03 22:14:01 浏览: 19
好的,下面是使用python tkinter开发俄罗斯方块游戏的代码。在代码中,我们使用了tkinter中的Canvas、Label、Button、Toplevel等组件来实现游戏界面和弹出式对话框。
```python
import tkinter as tk
import random
# 定义方块的种类和颜色
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
colors = ['red', 'orange', 'yellow', 'green', 'blue', 'purple', 'pink']
class Tetris:
def __init__(self, master):
self.width = 10
self.height = 20
self.cell_size = 30
self.master = master
self.canvas = tk.Canvas(master, width=self.width*self.cell_size, height=self.height*self.cell_size, bg='white')
self.canvas.pack()
self.current_shape = None
self.current_pos = None
self.current_color = None
self.score = 0
self.score_label = tk.Label(master, text='Score: 0')
self.score_label.pack()
self.game_over = False
self.init_game()
self.canvas.bind_all('<Left>', self.move_left)
self.canvas.bind_all('<Right>', self.move_right)
self.canvas.bind_all('<Down>', self.move_down)
self.canvas.bind_all('<Up>', self.rotate)
def init_game(self):
self.grid = [[0]*self.width for _ in range(self.height)]
self.draw_grid()
self.new_shape()
self.update_score()
def draw_grid(self):
self.canvas.delete('grid')
for i in range(self.width):
x0, y0, x1, y1 = i*self.cell_size, 0, i*self.cell_size, self.height*self.cell_size
self.canvas.create_line(x0, y0, x1, y1, tag='grid')
for i in range(self.height):
x0, y0, x1, y1 = 0, i*self.cell_size, self.width*self.cell_size, i*self.cell_size
self.canvas.create_line(x0, y0, x1, y1, tag='grid')
def new_shape(self):
shape_index = random.randint(0, len(shapes)-1)
self.current_shape = shapes[shape_index]
self.current_color = colors[shape_index]
self.current_pos = [self.width//2-len(self.current_shape[0])//2, 0]
if not self.is_valid_position(self.current_shape, self.current_pos):
self.game_over = True
self.show_game_over_dialog()
return
self.draw_shape(self.current_shape, self.current_pos, self.current_color)
def draw_shape(self, shape, pos, color):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] > 0:
x, y = pos[0]+j, pos[1]+i
self.draw_cell(x, y, color)
def erase_shape(self, shape, pos):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] > 0:
x, y = pos[0]+j, pos[1]+i
self.erase_cell(x, y)
def draw_cell(self, x, y, color):
x0, y0, x1, y1 = x*self.cell_size, y*self.cell_size, (x+1)*self.cell_size, (y+1)*self.cell_size
self.canvas.create_rectangle(x0, y0, x1, y1, fill=color, outline='white')
def erase_cell(self, x, y):
x0, y0, x1, y1 = x*self.cell_size, y*self.cell_size, (x+1)*self.cell_size, (y+1)*self.cell_size
self.canvas.create_rectangle(x0, y0, x1, y1, fill='white', outline='white')
def move_left(self, event):
if not self.game_over and self.is_valid_position(self.current_shape, [self.current_pos[0]-1, self.current_pos[1]]):
self.erase_shape(self.current_shape, self.current_pos)
self.current_pos[0] -= 1
self.draw_shape(self.current_shape, self.current_pos, self.current_color)
def move_right(self, event):
if not self.game_over and self.is_valid_position(self.current_shape, [self.current_pos[0]+1, self.current_pos[1]]):
self.erase_shape(self.current_shape, self.current_pos)
self.current_pos[0] += 1
self.draw_shape(self.current_shape, self.current_pos, self.current_color)
def move_down(self, event):
if not self.game_over and self.is_valid_position(self.current_shape, [self.current_pos[0], self.current_pos[1]+1]):
self.erase_shape(self.current_shape, self.current_pos)
self.current_pos[1] += 1
self.draw_shape(self.current_shape, self.current_pos, self.current_color)
else:
self.freeze_shape()
self.clear_rows()
self.new_shape()
def rotate(self, event):
if not self.game_over:
new_shape = [[self.current_shape[j][i] for j in range(len(self.current_shape))] for i in range(len(self.current_shape[0])-1, -1, -1)]
if self.is_valid_position(new_shape, self.current_pos):
self.erase_shape(self.current_shape, self.current_pos)
self.current_shape = new_shape
self.draw_shape(self.current_shape, self.current_pos, self.current_color)
def freeze_shape(self):
for i in range(len(self.current_shape)):
for j in range(len(self.current_shape[0])):
if self.current_shape[i][j] > 0:
x, y = self.current_pos[0]+j, self.current_pos[1]+i
self.grid[y][x] = self.current_shape[i][j]
def clear_rows(self):
rows_cleared = 0
for i in range(self.height-1, -1, -1):
if all(self.grid[i]):
rows_cleared += 1
for j in range(self.width):
self.erase_cell(j, i)
del self.grid[i]
self.grid.insert(0, [0]*self.width)
if rows_cleared > 0:
self.score += rows_cleared*10
self.update_score()
def update_score(self):
self.score_label.config(text='Score: {}'.format(self.score))
def is_valid_position(self, shape, pos):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] > 0:
x, y = pos[0]+j, pos[1]+i
if x < 0 or x >= self.width or y < 0 or y >= self.height or self.grid[y][x] > 0:
return False
return True
def show_game_over_dialog(self):
dialog = tk.Toplevel()
dialog.title('Game Over')
dialog.geometry('200x100')
message = tk.Label(dialog, text='Game Over!', font=('Helvetica', 18))
message.pack(pady=20)
button = tk.Button(dialog, text='Quit', command=self.master.quit)
button.pack()
root = tk.Tk()
root.title('Tetris')
game = Tetris(root)
root.mainloop()
```
在运行代码后,会弹出一个俄罗斯方块游戏的窗口,按照要求使用左、右和下箭头来控制方块的左、右和向下移动,使用上箭头来改变方块的方向。当游戏结束时会弹出一个弹出式对话框。
相关推荐
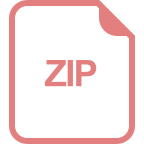
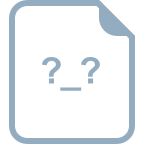












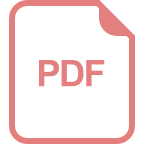
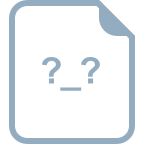
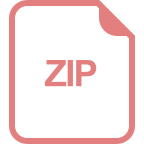