创建函数模板1.创建Student(数据成员string name ,int grade)类及所需函数。2.求出集合中的最大值,支持int,double,char,Student等类型的数据。3.排序方法,支持int,double, char,Student (按成绩排序) 等类型的数据。4.中位数指的是一组数,如果按照大小排序排好后最中间的那个数的值, 如果有偶数个元素,那么就是最中间两个数的平均数。求出集合中的中位数,支持int,double,Student集合中成绩的中位数。
时间: 2024-02-12 18:06:15 浏览: 20
好的,根据你的要求,我可以为你创建一个函数模板,实现以上功能。代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
template<typename T>
class Student {
public:
string name;
T grade;
Student(string n, T g) : name(n), grade(g) {}
bool operator<(const Student<T>& other) const {
return grade < other.grade;
}
};
template<typename T>
T findMax(const vector<T>& v) {
T maxVal = v[0];
for (const T& val : v) {
if (val > maxVal) {
maxVal = val;
}
}
return maxVal;
}
template<typename T>
void sortVector(vector<T>& v) {
sort(v.begin(), v.end());
}
template<typename T>
T findMedian(vector<T>& v) {
int n = v.size();
sortVector(v);
if (n % 2 == 0) {
return (v[n / 2 - 1] + v[n / 2]) / 2;
}
else {
return v[n / 2];
}
}
int main() {
vector<int> v1 = { 3, 5, 1, 7, 2, 8 };
cout << "Max value in v1: " << findMax(v1) << endl;
sortVector(v1);
cout << "Sorted v1: ";
for (const int& val : v1) {
cout << val << " ";
}
cout << endl;
cout << "Median value in v1: " << findMedian(v1) << endl;
vector<double> v2 = { 3.5, 5.2, 1.8, 7.1, 2.6, 8.9 };
cout << "Max value in v2: " << findMax(v2) << endl;
sortVector(v2);
cout << "Sorted v2: ";
for (const double& val : v2) {
cout << val << " ";
}
cout << endl;
cout << "Median value in v2: " << findMedian(v2) << endl;
vector<char> v3 = { 'c', 'a', 'b', 'e', 'd', 'f' };
cout << "Max value in v3: " << findMax(v3) << endl;
sortVector(v3);
cout << "Sorted v3: ";
for (const char& val : v3) {
cout << val << " ";
}
cout << endl;
cout << "Median value in v3: " << findMedian(v3) << endl;
vector<Student<int>> v4 = { Student<int>("Alice", 85), Student<int>("Bob", 75), Student<int>("Charlie", 90) };
cout << "Max value in v4: " << findMax(v4).grade << endl;
sortVector(v4);
cout << "Sorted v4: ";
for (const Student<int>& val : v4) {
cout << val.grade << " ";
}
cout << endl;
cout << "Median value in v4: " << findMedian(v4).grade << endl;
vector<Student<double>> v5 = { Student<double>("Alice", 85.5), Student<double>("Bob", 75.2), Student<double>("Charlie", 90.1) };
cout << "Max value in v5: " << findMax(v5).grade << endl;
sortVector(v5);
cout << "Sorted v5: ";
for (const Student<double>& val : v5) {
cout << val.grade << " ";
}
cout << endl;
cout << "Median value in v5: " << findMedian(v5).grade << endl;
return 0;
}
```
这是一个使用了函数模板的程序,包含了创建Student类及所需函数、求出集合中的最大值、排序方法以及中位数的计算。程序中的Student类是一个模板类,支持不同类型的成绩。findMax函数和sortVector函数都是模板函数,能够处理不同类型的数据。findMedian函数也是一个模板函数,能够处理包括int、double和Student类中成绩的中位数的计算。
阅读全文
相关推荐
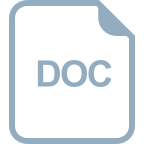
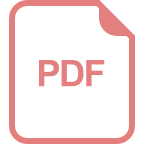
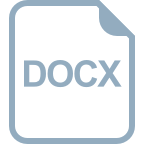














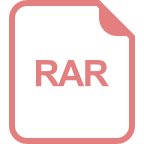
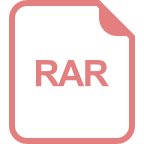