C++贪心算法实现异形排样程序
时间: 2023-07-17 09:56:19 浏览: 231
下面是一个使用贪心算法实现异形排样的C++示例程序:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct Rect {
int width;
int height;
bool isPlaced; // 是否已经放置
int x; // 左上角x坐标
int y; // 左上角y坐标
};
bool compareRect(const Rect& rect1, const Rect& rect2) {
// 按照面积从大到小排序
return rect1.width * rect1.height > rect2.width * rect2.height;
}
bool doesFit(const Rect& rect, int x, int y, int width, int height) {
// 检查物体是否适合给定的位置和大小
return (x + rect.width <= width) && (y + rect.height <= height);
}
void packRects(std::vector<Rect>& rects, int width, int height) {
// 按照面积从大到小排序
std::sort(rects.begin(), rects.end(), compareRect);
for (int i = 0; i < rects.size(); ++i) {
for (int j = 0; j < i; ++j) {
if (rects[j].isPlaced) {
// 尝试在已放置的矩形周围找到合适的位置
if (doesFit(rects[i], rects[j].x, rects[j].y + rects[j].height, width, height)) {
rects[i].x = rects[j].x;
rects[i].y = rects[j].y + rects[j].height;
rects[i].isPlaced = true;
break;
}
else if (doesFit(rects[i], rects[j].x + rects[j].width, rects[j].y, width, height)) {
rects[i].x = rects[j].x + rects[j].width;
rects[i].y = rects[j].y;
rects[i].isPlaced = true;
break;
}
}
}
if (!rects[i].isPlaced) {
// 如果没有找到合适的位置,则将物体放置在左上角
rects[i].x = 0;
rects[i].y = 0;
rects[i].isPlaced = true;
}
}
}
int main() {
int width = 100; // 区域宽度
int height = 100; // 区域高度
std::vector<Rect> rects = {
{20, 30, false, 0, 0},
{40, 50, false, 0, 0},
{30, 20, false, 0, 0},
{50, 40, false, 0, 0}
};
packRects(rects, width, height);
for (const auto& rect : rects) {
std::cout << "Width: " << rect.width << ", Height: " << rect.height << ", Position: (" << rect.x << ", " << rect.y << ")" << std::endl;
}
return 0;
}
```
上述程序中,我们定义了一个矩形结构体Rect,包含宽度、高度、是否已经放置、左上角坐标等信息。使用compareRect函数按照矩形面积从大到小进行排序,然后使用doesFit函数检查矩形是否适合给定的位置和大小。
在packRects函数中,我们首先对矩形进行排序,然后遍历每个矩形。对于每个矩形,我们尝试在已放置的矩形周围找到合适的位置,如果找到则更新矩形的位置和状态,否则将矩形放置在左上角。
最后,在主函数中我们定义了一个区域的宽度和高度,以及一组待排列的矩形。调用packRects函数进行异形排样,并输出每个矩形的宽度、高度和位置信息。
注意:上述代码只是一个简单的示例,实际应用中可能需要考虑更多的细节和优化。
阅读全文
相关推荐
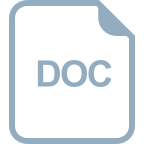

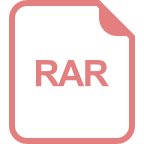

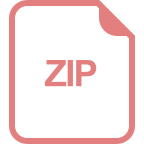
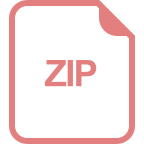
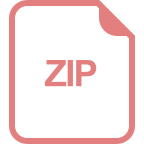
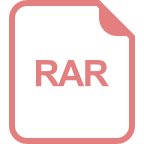
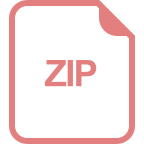
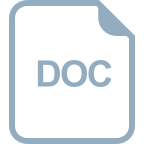



