使用Java将YAML转化成XML
时间: 2024-03-09 07:49:42 浏览: 49
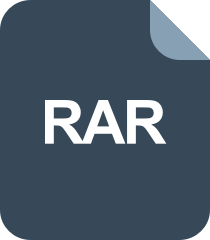
通过JAVA生成XML

以下是使用Java将YAML转换为XML的代码示例:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.constructor.Constructor;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import javax.xml.parsers.DocumentBuilderFactory;
public class YAMLtoXML {
public static void main(String[] args) {
try {
// 读取YAML文件
File file = new File("example.yaml");
FileInputStream input = new FileInputStream(file);
Constructor constructor = new Constructor();
Yaml yaml = new Yaml(constructor);
// 将YAML转换为Java对象
Object data = yaml.load(input);
// 将Java对象转换为XML
DocumentBuilderFactory docFactory = DocumentBuilderFactory.newInstance();
Document doc = docFactory.newDocumentBuilder().newDocument();
Element rootElement = doc.createElement("root");
doc.appendChild(rootElement);
for (Object key : ((Map<?, ?>) data).keySet()) {
Element element = doc.createElement(key.toString());
element.setTextContent(((Map<?, ?>) data).get(key).toString());
rootElement.appendChild(element);
}
// 将XML写入文件
FileWriter writer = new FileWriter("example.xml");
writer.write(docToString(doc));
writer.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static String docToString(Document doc) {
try {
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
StreamResult result = new StreamResult(new StringWriter());
DOMSource source = new DOMSource(doc);
transformer.transform(source, result);
return result.getWriter().toString();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
这个代码使用SnakeYAML库将YAML文件中的数据读取到一个Java对象中,然后使用Java标准库中的Document和Element类将数据转换为XML格式,并将结果写入文件。注意需要引入相关的库文件。
阅读全文
相关推荐















