BeginBatchDraw(); Start_time = clock(); while (1) { End_time = clock(); time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC); settextstyle(20, 0, "宋体"); setcolor(BLACK); sprintf_s(str, " %d s", time_1); outtextxy(10, 10, str); EndBatchDraw(); }加注释
时间: 2024-04-26 17:26:28 浏览: 8
这段代码使用了EasyX图形库,主要实现了一个计时功能。具体解释如下:
- BeginBatchDraw():开启绘图批处理模式,避免频繁刷新屏幕。
- Start_time = clock():记录程序开始执行时的CPU时钟计数值。
- while(1):无限循环。
- End_time = clock():获取当前的CPU时钟计数值。
- time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC):计算程序运行的秒数。
- settextstyle(20, 0, "宋体"):设置字体样式和大小。
- setcolor(BLACK):设置字体颜色为黑色。
- sprintf_s(str, " %d s", time_1):将计时的秒数转换成字符串,存储到str中。
- outtextxy(10, 10, str):在屏幕上绘制计时的秒数。
- EndBatchDraw():结束绘图批处理模式,将所有绘制的图形一次性输出到屏幕上。
相关问题
如何结束#include <stdio.h> #include <graphics.h> #include<time.h> clock_t Start_time; clock_t End_time; int time_1; char str[100]; int main() { initgraph(200, 200, SHOWCONSOLE);//init 初始化 graph 图像窗口 SHOWCONSOLE 显示控制台 setbkcolor(RGB(225, 115, 100));//设置背景颜色 BeginBatchDraw(); //双缓冲绘图,防止屏闪 Start_time = clock(); while (1) { cleardevice(); End_time = clock(); time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC); settextstyle(20, 0, "宋体"); setcolor(BLACK);// sprintf_s(str, " %d s", time_1); outtextxy(10, 10, str); EndBatchDraw();//双缓冲绘图 } return 0; }
这段代码本身没有错误,可以正常运行。但是由于 while 循环中没有任何退出条件,所以程序会一直运行,无法结束。因此你需要添加一个退出循环的条件,以便程序可以正常结束。
下面是一个修改后的代码,添加了一个 ESC 键退出循环的条件:
```c++
#include <stdio.h>
#include <graphics.h>
#include <conio.h>
#include <time.h>
clock_t Start_time;
clock_t End_time;
int time_1;
char str[100];
int main() {
initgraph(200, 200, SHOWCONSOLE); // init 初始化 graph 图像窗口 SHOWCONSOLE 显示控制台
setbkcolor(RGB(225, 115, 100)); // 设置背景颜色
BeginBatchDraw(); // 双缓冲绘图,防止屏闪
Start_time = clock();
while (1) {
cleardevice();
End_time = clock();
time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC);
settextstyle(20, 0, "宋体");
setcolor(BLACK);
sprintf_s(str, " %d s", time_1);
outtextxy(10, 10, str);
EndBatchDraw(); // 双缓冲绘图
if (_kbhit() && _getch() == 27) { // 如果按下 ESC 键,则退出循环
break;
}
}
getch();
closegraph();
return 0;
}
```
在这个修改后的代码中,使用 _kbhit 函数检测是否有键盘输入,如果有,则使用 _getch 函数获取键盘输入的字符。如果获取到的字符是 ESC 键,则退出循环,程序正常结束。
#include <thread> void timerThread() { initgraph(200, 200, SHOWCONSOLE); setbkcolor(RGB(225, 115, 100)); BeginBatchDraw(); Start_time = clock(); while (1) { cleardevice(); End_time = clock(); time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC); settextstyle(20, 0, "宋体"); setcolor(BLACK); sprintf_s(str, " %d s", time_1); outtextxy(10, 10, str); EndBatchDraw(); } } int main() { // Run other loops here std::thread timer(timerThread); // Join the thread to wait for it to finish timer.join(); return 0; }如何停止计时
要停止计时,你需要在 timerThread() 函数中添加一个条件语句,以决定何时停止计时。例如,你可以添加一个 bool 类型的变量 isStopped,并在 while 循环中检查它的值。如果 isStopped 为真,则退出循环,停止计时。
以下是修改后的代码示例:
```c++
#include <thread>
void timerThread() {
initgraph(200, 200, SHOWCONSOLE);
setbkcolor(RGB(225, 115, 100));
BeginBatchDraw();
Start_time = clock();
bool isStopped = false; // 添加一个布尔变量
while (!isStopped) { // 只有当 isStopped 为假时才更新时间和绘制窗口
cleardevice();
End_time = clock();
time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC);
settextstyle(20, 0, "宋体");
setcolor(BLACK);
sprintf_s(str, " %d s", time_1);
outtextxy(10, 10, str);
EndBatchDraw();
}
}
int main() {
// Run other loops here
std::thread timer(timerThread);
// 在这里添加停止计时的代码
while (1) {
// 检查键盘输入
if (kbhit()) {
char ch = getch();
if (ch == 's' || ch == 'S') {
timer.detach(); // 分离线程
break; // 退出主循环,停止计时
}
}
Sleep(100);
}
return 0;
}
```
在上面的代码中,我们在主循环中添加了停止计时的代码。当用户按下 's' 键时,我们调用 timer.detach() 分离线程,并退出主循环,停止计时。在 timerThread() 函数中,只有当 isStopped 为假时才更新时间和绘制窗口。如果 isStopped 为真,则退出循环,停止计时。这样就可以实现停止计时的效果。
相关推荐
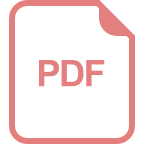
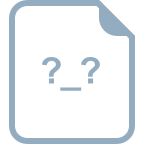









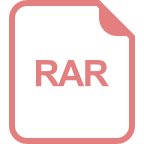
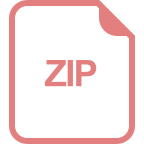
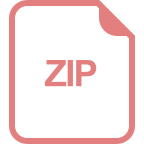