用python实现栅栏密码的加密和解密
时间: 2023-12-22 07:05:41 浏览: 267
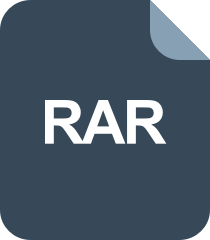
栅栏密码加密解密

栅栏密码是一种简单的替换密码,其基本思想是将明文中的字符沿着一个规定的路径排列,然后从上到下,从左到右依次读取。举个例子,如果明文是"HELLO WORLD",路径是3,那么加密后的密文就是"HOLWRLELD O"。
加密过程:
1. 将明文按照规定的路径排列成矩阵。
2. 按照行优先的顺序读取矩阵中的字符,得到密文。
解密过程:
1. 根据密文长度和路径计算出矩阵的行数和列数。
2. 将密文按照列优先的顺序排列成矩阵。
3. 按照行优先的顺序读取矩阵中的字符,得到明文。
下面是用Python实现栅栏密码的加密和解密:
```python
def encrypt(plain_text, path):
# 将明文按照路径排列成矩阵
rows = len(plain_text) // path
if len(plain_text) % path != 0:
rows += 1
matrix = [[''] * path for i in range(rows)]
index = 0
for i in range(rows):
for j in range(path):
if index < len(plain_text):
matrix[i][j] = plain_text[index]
index += 1
else:
break
# 按行优先顺序读取矩阵中的字符,得到密文
cipher_text = ''
for j in range(path):
for i in range(rows):
cipher_text += matrix[i][j]
return cipher_text
def decrypt(cipher_text, path):
# 根据密文长度和路径计算出矩阵的行数和列数
rows = len(cipher_text) // path
if len(cipher_text) % path != 0:
rows += 1
# 将密文按照列优先的顺序排列成矩阵
matrix = [[''] * rows for i in range(path)]
index = 0
for j in range(path):
for i in range(rows):
if index < len(cipher_text):
matrix[j][i] = cipher_text[index]
index += 1
else:
break
# 按照行优先的顺序读取矩阵中的字符,得到明文
plain_text = ''
for i in range(rows):
for j in range(path):
plain_text += matrix[j][i]
return plain_text
```
下面是一个示例:
```python
plain_text = 'HELLO WORLD'
path = 3
cipher_text = encrypt(plain_text, path)
print(cipher_text)
plain_text2 = decrypt(cipher_text, path)
print(plain_text2)
```
输出结果:
```
HOLWRLELD O
HELLO WORLD
```
阅读全文
相关推荐
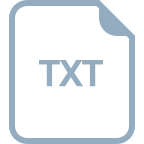
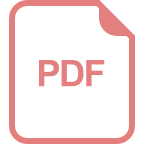








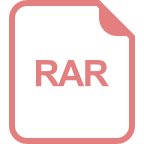
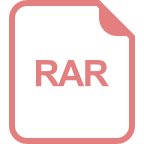
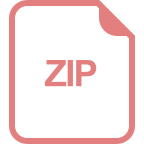


