给出以下代码的注释bool AStarExpansion::calculatePotentials(unsigned char* costs, double start_x, double start_y, double end_x, double end_y, int cycles, float* potential) { queue_.clear(); int start_i = toIndex(start_x, start_y); queue_.push_back(Index(start_i, 0)); std::fill(potential, potential + ns_, POT_HIGH); potential[start_i] = 0; int goal_i = toIndex(end_x, end_y); int cycle = 0; while (queue_.size() > 0 && cycle < cycles) { Index top = queue_[0]; std::pop_heap(queue_.begin(), queue_.end(), greater1()); queue_.pop_back(); int i = top.i; if (i == goal_i) return true; add(costs, potential, potential[i], i + 1, end_x, end_y); add(costs, potential, potential[i], i - 1, end_x, end_y); add(costs, potential, potential[i], i + nx_, end_x, end_y); add(costs, potential, potential[i], i - nx_, end_x, end_y); cycle++; } return false; }
时间: 2024-03-31 19:37:09 浏览: 51
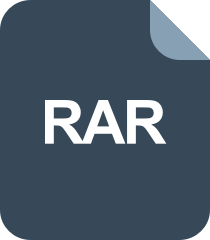
double_cache.rar_VC 双缓冲
这段代码是一个 A* 算法的实现,用于计算一个地图上的各个点到目标点的最短路径。其中,参数 costs 是一个数组,表示地图上各个点的代价;start_x 和 start_y 分别是起点的 x、y 坐标;end_x 和 end_y 分别是终点的 x、y 坐标;cycles 表示算法运行的最大迭代次数;potential 是一个数组,用于存储各个点到目标点的代价估计值。算法的具体实现过程是:首先将起点加入到队列中,并初始化 potential 数组;然后从队列中取出距离目标点最近的点,计算它的相邻点的 potential 值,并将它们加入到队列中;重复上述过程,直到找到终点或者达到最大迭代次数。函数返回一个布尔值,表示是否找到了最短路径。
阅读全文
相关推荐
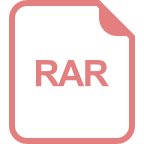
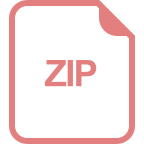







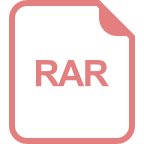
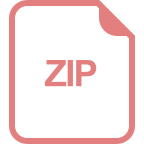





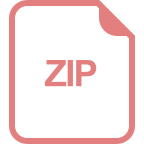