bool IfLinesIntersect(Point2D line1_start, Point2D line1_end, Point2D line2_start, Point2D line2_end)
时间: 2024-03-20 17:41:02 浏览: 54
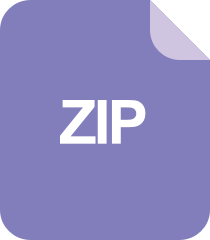
line-segments-intersect:确定两个线段是否相交
这是一个判断两条线段是否相交的函数,可以用于解决线段相交问题:
```c++
struct Point2D {
double x, y;
};
// 判断两条线段是否相交
bool IfLinesIntersect(Point2D line1_start, Point2D line1_end, Point2D line2_start, Point2D line2_end) {
// 计算向量叉积
auto crossProduct = [](Point2D a, Point2D b, Point2D c) -> double {
return (b.x - a.x) * (c.y - a.y) - (b.y - a.y) * (c.x - a.x);
};
// 判断两条线段是否相交
double c1 = crossProduct(line1_start, line1_end, line2_start);
double c2 = crossProduct(line1_start, line1_end, line2_end);
double c3 = crossProduct(line2_start, line2_end, line1_start);
double c4 = crossProduct(line2_start, line2_end, line1_end);
if (((c1 > 0 && c2 < 0) || (c1 < 0 && c2 > 0)) && ((c3 > 0 && c4 < 0) || (c3 < 0 && c4 > 0))) {
return true;
}
if (c1 == 0 && line2_start.x >= min(line1_start.x, line1_end.x) && line2_start.x <= max(line1_start.x, line1_end.x) && line2_start.y >= min(line1_start.y, line1_end.y) && line2_start.y <= max(line1_start.y, line1_end.y)) {
return true;
}
if (c2 == 0 && line2_end.x >= min(line1_start.x, line1_end.x) && line2_end.x <= max(line1_start.x, line1_end.x) && line2_end.y >= min(line1_start.y, line1_end.y) && line2_end.y <= max(line1_start.y, line1_end.y)) {
return true;
}
if (c3 == 0 && line1_start.x >= min(line2_start.x, line2_end.x) && line1_start.x <= max(line2_start.x, line2_end.x) && line1_start.y >= min(line2_start.y, line2_end.y) && line1_start.y <= max(line2_start.y, line2_end.y)) {
return true;
}
if (c4 == 0 && line1_end.x >= min(line2_start.x, line2_end.x) && line1_end.x <= max(line2_start.x, line2_end.x) && line1_end.y >= min(line2_start.y, line2_end.y) && line1_end.y <= max(line2_start.y, line2_end.y)) {
return true;
}
return false;
}
```
其中,向量叉积的计算公式为:
$$(x2-x1) \times (y3-y1) - (y2-y1) \times (x3-x1)$$
如果结果为正数,则表示向量 $\vec{AB}$ 在向量 $\vec{AC}$ 的顺时针方向,即 $C$ 在 $AB$ 的右侧。如果结果为负数,则表示向量 $\vec{AB}$ 在向量 $\vec{AC}$ 的逆时针方向,即 $C$ 在 $AB$ 的左侧。如果结果为 $0$,则表示 $A$、$B$、$C$ 三点共线。
在判断两条线段是否相交时,我们需要计算四个向量的叉积,如果满足如下条件,则两条线段相交:
1. 向量 $\vec{AB}$ 与向量 $\vec{AC}$ 在向量 $\vec{AB}$ 的两侧,即 $C$ 在 $AB$ 的右侧,$D$ 在 $AB$ 的左侧。
2. 向量 $\vec{CD}$ 与向量 $\vec{CA}$ 在向量 $\vec{CD}$ 的两侧,即 $A$ 在 $CD$ 的右侧,$B$ 在 $CD$ 的左侧。
3. 向量 $\vec{AB}$ 与向量 $\vec{AC}$ 在同侧,即 $C$ 在 $AB$ 的左侧,$D$ 在 $AB$ 的左侧。
4. 向量 $\vec{CD}$ 与向量 $\vec{CA}$ 在同侧,即 $A$ 在 $CD$ 的左侧,$B$ 在 $CD$ 的左侧。
如果以上条件都不满足,则两条线段不相交。
阅读全文
相关推荐
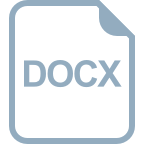
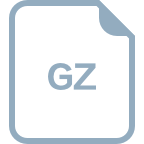
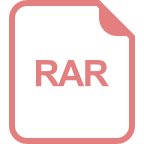
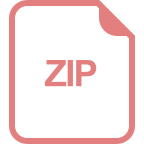
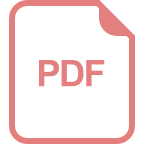
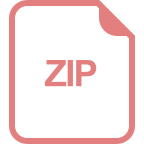
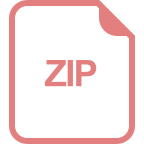
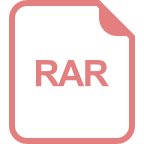
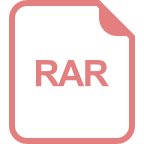
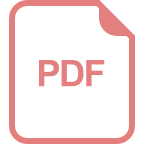
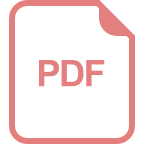
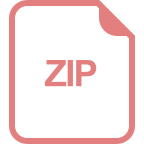
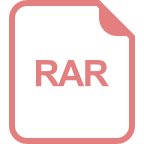
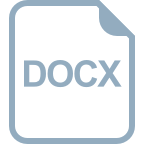
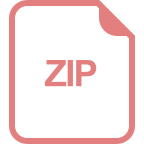
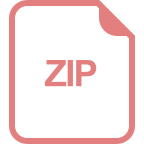
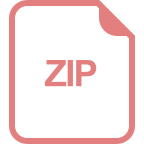
