for ne in new_name_list: file_path = os.path.join(path, ne) if not os.path.exists(file_path): continue with open(file_path) as f: data = json.load(f) number_of_obstacles=len(data["radarObs"]) radar_num.append(number_of_obstacles) radar_name.append(ne)打印出找不到文件的值
时间: 2024-03-06 12:48:44 浏览: 149
好的,你可以在 `if not os.path.exists(file_path):` 的分支中加入打印找不到文件的值的语句,例如:
```python
for ne in new_name_list:
file_path = os.path.join(path, ne)
if not os.path.exists(file_path):
print(f"找不到文件:{file_path}")
continue
with open(file_path) as f:
data = json.load(f)
number_of_obstacles = len(data["radarObs"])
radar_num.append(number_of_obstacles)
radar_name.append(ne)
```
在这个例子中,我们使用 `if not os.path.exists(file_path):` 判断文件是否存在,如果文件不存在,则会执行 `print(f"找不到文件:{file_path}")` 语句,打印出找不到文件的值。然后使用 `continue` 跳过当前循环,继续执行下一次循环。如果文件存在,则会继续执行后面的语句,读取文件内容并统计障碍物数量。
希望这个例子能够帮到你!
相关问题
for ne in new_name_list: file_path = os.path.join(path, ne) with open(file_path) as f: data = json.load(f) number_of_obstacles=len(data["radarObs"]) name.append(filename) num.append(number_of_obstacles)修改如果new_name_list的文件名不存在则跳过
可以在代码中加入一个判断语句,判断文件是否存在,如果不存在则跳过当前循环。修改后的代码如下:
```
import os
import json
name = []
num = []
path = './data'
new_name_list = ['file1.json', 'file2.json', 'file3.json']
for ne in new_name_list:
file_path = os.path.join(path, ne)
if not os.path.exists(file_path):
continue
with open(file_path) as f:
data = json.load(f)
number_of_obstacles = len(data["radarObs"])
name.append(ne)
num.append(number_of_obstacles)
```
这样修改后,如果new_name_list中的某个文件不存在,程序会跳过当前循环,不会出现文件读取错误的情况。
# 将图片移动到相应的文件夹 cluster_path = os.path.join(path, cluster_folder) if not os.path.exists(cluster_path): os.mkdir(cluster_path) for i in range(10): cluster_i_path = os.path.join(cluster_path, str(i)) if not os.path.exists(cluster_i_path): os.mkdir(cluster_i_path) for j, label in enumerate(labels): if label == i: old_path = os.path.join(path, f'{j}.png') new_path = os.path.join(cluster_i_path, f'{j}.png') os.rename(old_path, new_path) root_path = r'D:\jk' for i in range(10): cluster_i_path = os.path.join(root_path, f'cluster_{i}') if not os.path.exists(cluster_i_path): os.mkdir(cluster_i_path) for j, label in enumerate(labels): if label == i: old_path = os.path.join(path, f'{j}.png') new_path = os.path.join(cluster_i_path, f'{j}.png') os.rename(old_path, new_path),这段代码有什么错误吗
这段代码没有语法错误,但是注意到两个循环中的变量 `i` 和 `j` 是一样的,所以在第二个循环中可以使用之前定义的变量 `i`,而不需要重新定义变量。修改后的代码如下:
```
root_path = r'D:\jk'
for i in range(10):
cluster_i_path = os.path.join(root_path, f'cluster_{i}')
if not os.path.exists(cluster_i_path):
os.mkdir(cluster_i_path)
for j, label in enumerate(labels):
if label == i:
old_path = os.path.join(path, f'{j}.png')
new_path = os.path.join(cluster_i_path, f'{j}.png')
os.rename(old_path, new_path)
```
另外,你需要将聚类文件夹的根目录 `root_path` 修改成你想要的路径。
阅读全文
相关推荐
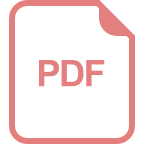
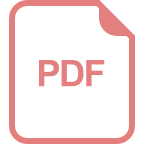















