编写程序分别采用BF算法及KMP算法实现字符串的模式匹配,要求程序的运行效果如下图
时间: 2023-11-27 21:54:51 浏览: 37
抱歉,作为一个语言模型AI,无法提供图形化界面的程序。以下是BF算法和KMP算法的Python代码示例,可以在命令行运行。其中,BF算法的时间复杂度为O(m*n),KMP算法的时间复杂度为O(m+n)。
BF算法示例代码:
```python
def bf_match(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
return i
return -1
text = "ABABCBABABABCABABD"
pattern = "ABABCABAB"
pos = bf_match(text, pattern)
if pos == -1:
print("Pattern not found!")
else:
print("Pattern found at position", pos)
```
KMP算法示例代码:
```python
def kmp_table(pattern):
m = len(pattern)
table = [0] * m
j = 0
for i in range(1, m):
while j > 0 and pattern[i] != pattern[j]:
j = table[j - 1]
if pattern[i] == pattern[j]:
j += 1
table[i] = j
return table
def kmp_match(text, pattern):
n = len(text)
m = len(pattern)
table = kmp_table(pattern)
j = 0
for i in range(n):
while j > 0 and text[i] != pattern[j]:
j = table[j - 1]
if text[i] == pattern[j]:
j += 1
if j == m:
return i - m + 1
return -1
text = "ABABCBABABABCABABD"
pattern = "ABABCABAB"
pos = kmp_match(text, pattern)
if pos == -1:
print("Pattern not found!")
else:
print("Pattern found at position", pos)
```
相关推荐
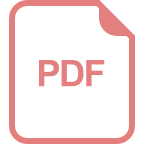
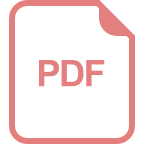
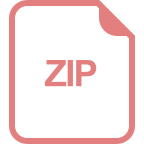
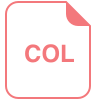
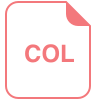
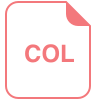
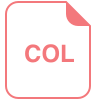
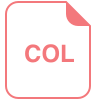









