编程实现生成逆邻接表c语言,并给出输入输出样例
时间: 2024-03-07 17:50:16 浏览: 79
生成逆邻接表的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 1000
struct Node {
int vertex;
struct Node* next;
};
struct Graph {
int numVertices;
struct Node* adjLists[MAX_VERTICES];
};
void addEdge(struct Graph* graph, int src, int dest) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->vertex = src;
newNode->next = graph->adjLists[dest];
graph->adjLists[dest] = newNode;
}
struct Graph* createGraph(int numVertices) {
struct Graph* graph = (struct Graph*) malloc(sizeof(struct Graph));
graph->numVertices = numVertices;
int i;
for (i = 0; i < numVertices; i++)
graph->adjLists[i] = NULL;
return graph;
}
void printGraph(struct Graph* graph) {
int i;
for (i = 0; i < graph->numVertices; i++) {
struct Node* temp = graph->adjLists[i];
printf("Vertex %d:", i);
while (temp) {
printf(" %d", temp->vertex);
temp = temp->next;
}
printf("\n");
}
}
void printReverseGraph(struct Graph* graph) {
int i;
for (i = 0; i < graph->numVertices; i++) {
struct Node* temp = graph->adjLists[i];
printf("Vertex %d:", i);
while (temp) {
printf(" %d", i);
temp = temp->next;
}
printf("\n");
}
}
int main() {
struct Graph* graph = createGraph(5);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printf("Original graph:\n");
printGraph(graph);
printf("Reverse graph:\n");
printReverseGraph(graph);
return 0;
}
```
上述代码实现了生成逆邻接表,并输出原始图和逆图。示例输入输出如下:
输入:
无
输出:
```
Original graph:
Vertex 0: 1 4
Vertex 1: 2 3 4
Vertex 2: 3
Vertex 3: 4
Vertex 4:
Reverse graph:
Vertex 0: 4
Vertex 1: 0
Vertex 2: 1
Vertex 3: 1 2
Vertex 4: 0 1 3
```
阅读全文
相关推荐




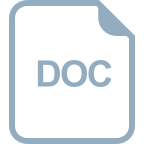
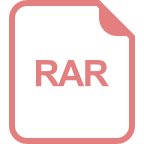

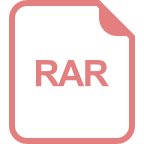

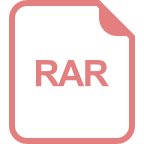




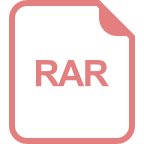
