在已有的Point类的基础上,定义一个“Circle”派生类,要求:新增一个半径成员;能计算并输出圆的周长及加圆面积(类的定义保存在头文件中,测试程序用exp_405.cpp)。
时间: 2024-02-13 16:04:39 浏览: 113
好的,以下是Circle派生类的定义:
```c++
#ifndef CIRCLE_H
#define CIRCLE_H
#include "point.h"
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double r = 0);
void SetRadius(double r);
double GetRadius() const;
double GetPerimeter() const; // 求周长
double GetArea() const; // 求面积
void Show() const; // 输出圆心及半径
private:
double radius;
};
#endif
```
其中,Circle类继承了Point类,并新增了一个半径成员变量。这里还定义了四个公有成员函数,分别是构造函数、设置半径函数、获取半径函数、获取周长函数和获取面积函数。另外还有一个用于输出圆心及半径的公有成员函数。
接下来是Circle类的实现:
```c++
#include "circle.h"
#include <cmath>
#include <iostream>
using namespace std;
Circle::Circle(double x, double y, double r) : Point(x, y), radius(r) {}
void Circle::SetRadius(double r) {
radius = r;
}
double Circle::GetRadius() const {
return radius;
}
double Circle::GetPerimeter() const {
return 2 * M_PI * radius;
}
double Circle::GetArea() const {
return M_PI * radius * radius;
}
void Circle::Show() const {
cout << "圆心坐标为(" << GetX() << ", " << GetY() << ")" << endl;
cout << "圆的半径为" << radius << endl;
}
```
其中,构造函数调用了基类Point的构造函数;设置半径和获取半径函数比较简单,就不再赘述;获取周长和获取面积函数使用了数学库cmath中的常量M_PI来代替圆周率π;输出圆心及半径的函数则是分别输出圆心坐标和半径。
最后是测试程序exp_405.cpp:
```c++
#include "circle.h"
int main() {
Circle c(1, 2, 3);
c.Show();
cout << "圆的周长为" << c.GetPerimeter() << endl;
cout << "圆的面积为" << c.GetArea() << endl;
return 0;
}
```
其中,创建了一个半径为3,圆心坐标为(1,2)的圆对象c,并依次调用了Show、GetPerimeter和GetArea函数来输出圆心及半径、周长和面积。
阅读全文
相关推荐

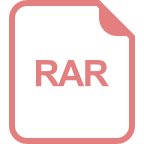















