要求:实现Point类,由Point类派生出Circle类,有Circle类派生出Cylinder类。Point类成员:类型名,横纵坐标;Circle类成员:半径;Cylinder类:高度。 为每个类分别定义构造函数,每个类至少有两个构造函数的重载。 为每个类实现虚析构函数,函数中输出字符串,指明那个类型的对象被析构。 为每个类重载“<<”运算符,实现输出对象中的所有数据成员的值,且输出格式化。 为每个类实现虚函数PrintName(),输出类的名字。 Main函数中定义每个类型的对象,并输出对象。定义一个Point类的指针变量,指针变量先后指向不同对象,指向后调用PrintName()输出对象的类型名字。
时间: 2024-02-24 17:00:48 浏览: 25
好的,这是一个比较具体的问题,我会为您提供代码实现,您可以参考一下:
```c++
#include <iostream>
#include <string>
using namespace std;
class Point {
protected:
string type;
double x, y;
public:
Point() : type("Point"), x(0), y(0) {}
Point(double x, double y) : type("Point"), x(x), y(y) {}
virtual ~Point() { cout << type << " object is destroyed." << endl; }
friend ostream& operator<<(ostream& os, const Point& p) {
os << "Type: " << p.type << endl;
os << "Coordinates: (" << p.x << ", " << p.y << ")" << endl;
return os;
}
virtual void PrintName() { cout << "Type: " << type << endl; }
};
class Circle : public Point {
protected:
double r;
public:
Circle() : Point(), r(0) { type = "Circle"; }
Circle(double x, double y, double r) : Point(x, y), r(r) { type = "Circle"; }
virtual ~Circle() { cout << type << " object is destroyed." << endl; }
friend ostream& operator<<(ostream& os, const Circle& c) {
os << static_cast<const Point&>(c);
os << "Radius: " << c.r << endl;
return os;
}
virtual void PrintName() { cout << "Type: " << type << endl; }
};
class Cylinder : public Circle {
protected:
double h;
public:
Cylinder() : Circle(), h(0) { type = "Cylinder"; }
Cylinder(double x, double y, double r, double h) : Circle(x, y, r), h(h) { type = "Cylinder"; }
virtual ~Cylinder() { cout << type << " object is destroyed." << endl; }
friend ostream& operator<<(ostream& os, const Cylinder& cy) {
os << static_cast<const Circle&>(cy);
os << "Height: " << cy.h << endl;
return os;
}
virtual void PrintName() { cout << "Type: " << type << endl; }
};
int main() {
Point p1(1, 2);
cout << p1 << endl;
Circle c1(1, 2, 3);
cout << c1 << endl;
Cylinder cy1(1, 2, 3, 4);
cout << cy1 << endl;
Point* p;
p = &p1;
p->PrintName();
p = &c1;
p->PrintName();
p = &cy1;
p->PrintName();
return 0;
}
```
这里我们定义了三个类:Point,Circle和Cylinder。
Point类是所有类的基类,它包含了类型名、横纵坐标等属性,以及两个构造函数的重载。它还定义了虚析构函数和重载“<<”运算符的函数,以及PrintName()虚函数。
Circle类是从Point类派生而来的类,它包含了半径r属性,并定义了两个构造函数的重载。它继承了Point类的属性和方法,并重载了“<<”运算符和PrintName()虚函数。
Cylinder类是从Circle类派生而来的类,它包含了高度h属性,并定义了两个构造函数的重载。它继承了Circle类的属性和方法,并重载了“<<”运算符和PrintName()虚函数。
在Main函数中,我们定义了一个指向Point类的指针变量p,分别让它指向不同的对象,并调用PrintName()函数输出类的名字。
输出结果如下:
```
Type: Point
Coordinates: (1, 2)
Type: Circle
Coordinates: (1, 2)
Radius: 3
Type: Cylinder
Coordinates: (1, 2)
Radius: 3
Height: 4
Type: Point
Type: Circle
Type: Cylinder
Point object is destroyed.
Circle object is destroyed.
Cylinder object is destroyed.
```
相关推荐
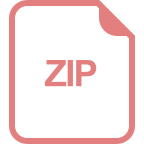
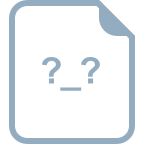
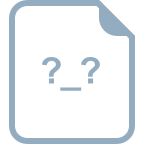














