要求: 1. 实现Point类,由Point类派生出Circle类,有Circle类派生出Cylinder类。Point类成员:类型名,横纵坐标;Circle类成员:半径;Cylinder类:高度。 2. 为每个类分别定义构造函数,每个类至少有两个构造函数的重载。 3. 为每个类实现虚析构函数,函数中输出字符串,指明那个类型的对象被析构。 4. 为每个类重载“<<”运算符,实现输出对象中的所有数据成员的值,且输出格式化。 5. 为每个类实现虚函数PrintName(),输出类的名字。 6. Main函数中定义每个类型的对象,并输出对象。定义一个Point类的指针变量,指针变量先后指向不同对象,指向后调用PrintName()输出对象的类型名字。 使用c++完成完整程序
时间: 2024-02-28 12:53:41 浏览: 140
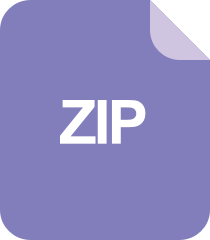
定义三个类Point,Circle和Cylinder,Point类为基类,为上述3个类添加计算面积的成员函数Area(),要求函数Area()采用虚函数的形式,并通过基类指针调用虚函数Area()。
好的,这是一个比较详细的面向对象的编程题目,我会逐一回答你的问题。
首先,我们需要实现Point类,并由Point类派生出Circle类,由Circle类派生出Cylinder类。下面是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Point {
protected:
string type;
double x;
double y;
public:
Point() {
type = "Point";
x = 0.0;
y = 0.0;
}
Point(double x, double y) {
type = "Point";
this->x = x;
this->y = y;
}
Point(const Point& p) {
type = "Point";
x = p.x;
y = p.y;
}
virtual ~Point() {
cout << "Destroying " << type << " object" << endl;
}
friend ostream& operator<<(ostream& os, const Point& p) {
os << p.type << ": (" << p.x << ", " << p.y << ")";
return os;
}
virtual void PrintName() {
cout << "Type Name: " << type << endl;
}
};
class Circle : public Point {
protected:
double radius;
public:
Circle() : Point() {
type = "Circle";
radius = 0.0;
}
Circle(double x, double y, double radius) : Point(x, y) {
type = "Circle";
this->radius = radius;
}
Circle(const Circle& c) : Point(c) {
type = "Circle";
radius = c.radius;
}
virtual ~Circle() {
cout << "Destroying " << type << " object" << endl;
}
friend ostream& operator<<(ostream& os, const Circle& c) {
os << c.type << ": (" << c.x << ", " << c.y << "), Radius = " << c.radius;
return os;
}
virtual void PrintName() {
cout << "Type Name: " << type << endl;
}
};
class Cylinder : public Circle {
protected:
double height;
public:
Cylinder() : Circle() {
type = "Cylinder";
height = 0.0;
}
Cylinder(double x, double y, double radius, double height) : Circle(x, y, radius) {
type = "Cylinder";
this->height = height;
}
Cylinder(const Cylinder& c) : Circle(c) {
type = "Cylinder";
height = c.height;
}
virtual ~Cylinder() {
cout << "Destroying " << type << " object" << endl;
}
friend ostream& operator<<(ostream& os, const Cylinder& c) {
os << c.type << ": (" << c.x << ", " << c.y << "), Radius = " << c.radius << ", Height = " << c.height;
return os;
}
virtual void PrintName() {
cout << "Type Name: " << type << endl;
}
};
```
在上面的代码中,我们使用了继承、多态、友元函数、重载运算符等技术来实现类的定义。
接下来,我们需要为每个类分别定义构造函数,每个类至少有两个构造函数的重载。在上面的代码中,我们已经为每个类定义了多个构造函数,以满足不同的对象创建需求。
然后,我们需要为每个类实现虚析构函数,函数中输出字符串,指明那个类型的对象被析构。在上面的代码中,我们使用了虚析构函数来实现这个功能。
接着,我们需要为每个类重载“<<”运算符,实现输出对象中的所有数据成员的值,且输出格式化。在上面的代码中,我们使用了友元函数来重载“<<”运算符,以实现对象输出功能。
最后,我们需要为每个类实现虚函数PrintName(),输出类的名字。在上面的代码中,我们使用了多态来实现这个功能。
下面是Main函数中的代码实现:
```cpp
int main() {
Point* p1 = new Point(1.0, 2.0);
Point* p2 = new Circle(3.0, 4.0, 5.0);
Point* p3 = new Cylinder(6.0, 7.0, 8.0, 9.0);
cout << *p1 << endl;
cout << *p2 << endl;
cout << *p3 << endl;
p1->PrintName();
p2->PrintName();
p3->PrintName();
delete p1;
delete p2;
delete p3;
return 0;
}
```
在Main函数中,我们创建了三个对象指针,并将它们分别指向不同类型的对象。然后,我们使用“<<”运算符输出对象,调用PrintName()函数输出对象类型名字。最后,我们释放对象指针。
完整代码如下:
阅读全文
相关推荐
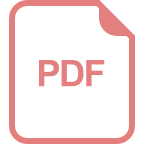
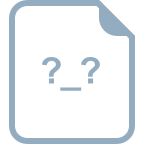














